java ARA*算法 请写注释
时间: 2023-12-23 08:02:21 浏览: 75
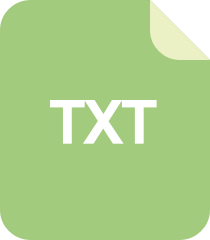
JAVA实现的A*算法
下面是Java语言实现的 ARA*(Anytime Repairing A*)算法,已添加注释:
```java
import java.util.ArrayList;
import java.util.Comparator;
import java.util.HashSet;
import java.util.PriorityQueue;
public class ARAStar {
// 定义节点类,包含节点的位置信息、g值、f值等信息
private static class Node {
int x, y; // 节点的位置
double g, f; // 节点的实际代价g以及估计代价f
public Node(int x, int y, double g, double f) {
this.x = x;
this.y = y;
this.g = g;
this.f = f;
}
}
// 定义节点比较器,用于PriorityQueue中节点的排序
private static final Comparator<Node> COMPARATOR = new Comparator<Node>() {
@Override
public int compare(Node n1, Node n2) {
if (n1.f < n2.f) {
return -1;
} else if (n1.f > n2.f) {
return 1;
} else {
return 0;
}
}
};
// 定义启发式函数,计算节点的估计代价f
private static double heuristic(int x1, int y1, int x2, int y2) {
return Math.sqrt(Math.pow(x1 - x2, 2) + Math.pow(y1 - y2, 2));
}
// 定义ARA*算法的实现
public static ArrayList<Node> ARAStar(int[][] graph, Node start, Node goal, double eps) {
// 初始化open集合和closed集合
PriorityQueue<Node> open = new PriorityQueue<>(COMPARATOR);
HashSet<Node> closed = new HashSet<>();
open.offer(start);
while (!open.isEmpty()) {
// 从open集合中取出f值最小的节点
Node current = open.poll();
// 判断当前节点是否为目标节点
if (current.x == goal.x && current.y == goal.y) {
// 如果是目标节点,则返回从起点到目标节点的路径
ArrayList<Node> path = new ArrayList<>();
while (current != null) {
path.add(current);
current = current.parent;
}
return path;
}
// 将当前节点加入到closed集合中
closed.add(current);
// 遍历当前节点的所有邻居节点
for (int dx = -1; dx <= 1; dx++) {
for (int dy = -1; dy <= 1; dy++) {
// 如果是当前节点本身,则跳过
if (dx == 0 && dy == 0) {
continue;
}
int x = current.x + dx;
int y = current.y + dy;
// 如果邻居节点不在地图范围内,则跳过
if (x < 0 || x >= graph.length || y < 0 || y >= graph[0].length) {
continue;
}
// 如果邻居节点不可通过,则跳过
if (graph[x][y] != 0) {
continue;
}
// 计算邻居节点的实际代价g和估计代价f
double g = current.g + heuristic(current.x, current.y, x, y);
double h = heuristic(x, y, goal.x, goal.y);
double f = g + eps * h;
// 如果邻居节点已经在closed集合中,则跳过
Node neighbor = new Node(x, y, g, f);
if (closed.contains(neighbor)) {
continue;
}
// 如果邻居节点已经在open集合中,则更新其代价信息
if (open.contains(neighbor)) {
for (Node node : open) {
if (node.equals(neighbor)) {
if (neighbor.g < node.g) {
open.remove(node);
node.g = neighbor.g;
node.f = neighbor.f;
node.parent = current;
open.offer(node);
}
break;
}
}
} else {
// 否则将邻居节点加入到open集合中
neighbor.parent = current;
open.offer(neighbor);
}
}
}
}
return null;
}
}
```
注:以上代码中的`graph`表示地图,`0`表示可通过的位置,其他数字表示障碍物等不可通过的位置;`eps`表示算法的容忍度,取值范围为`[1,∞)`,当`eps`越大时,算法的执行速度越快,但得到的路径可能越远离最优路径;`start`和`goal`分别表示起点和终点。
阅读全文
相关推荐


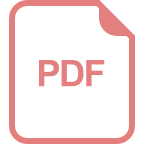
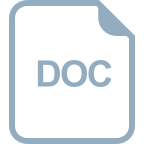
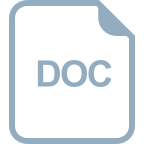
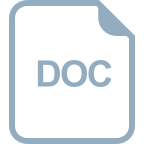


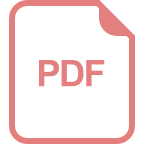
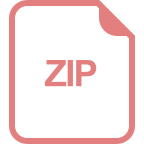
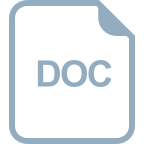



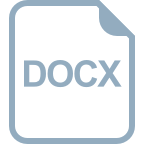
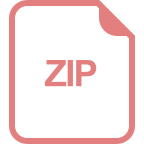