DQN实现坦克大战代码
时间: 2023-11-20 21:41:32 浏览: 204
DQN是深度强化学习算法,用于解决决策问题,如游戏中的行动选择。坦克大战是一个经典的游戏,可以用DQN来实现。
以下是使用Python和TensorFlow库实现DQN坦克大战游戏的代码:
1. 安装必要的库
```python
!pip install tensorflow==2.0.0
!pip install gym==0.17.2
!pip install gym[atari]
```
2. 导入库
```python
import gym
import random
import numpy as np
from collections import deque
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Conv2D, MaxPooling2D, Flatten
from tensorflow.keras.optimizers import Adam
```
3. 定义DQN的模型
```python
def build_model(state_shape, action_shape):
model = Sequential()
model.add(Conv2D(32, (8, 8), strides=(4, 4), activation='relu', input_shape=state_shape))
model.add(Conv2D(64, (4, 4), strides=(2, 2), activation='relu'))
model.add(Conv2D(64, (3, 3), strides=(1, 1), activation='relu'))
model.add(Flatten())
model.add(Dense(512, activation='relu'))
model.add(Dense(action_shape, activation='linear'))
model.compile(loss='mse', optimizer=Adam(lr=0.0001))
return model
```
4. 定义DQN的代理
```python
class DQNAgent:
def __init__(self, state_shape, action_shape):
self.state_shape = state_shape
self.action_shape = action_shape
self.memory = deque(maxlen=2000)
self.gamma = 0.95
self.epsilon = 1.0
self.epsilon_min = 0.01
self.epsilon_decay = 0.995
self.model = build_model(state_shape, action_shape)
def remember(self, state, action, reward, next_state, done):
self.memory.append((state, action, reward, next_state, done))
def act(self, state):
if np.random.rand() <= self.epsilon:
return random.randrange(self.action_shape)
q_values = self.model.predict(state)
return np.argmax(q_values[0])
def replay(self, batch_size):
if len(self.memory) < batch_size:
return
minibatch = random.sample(self.memory, batch_size)
for state, action, reward, next_state, done in minibatch:
target = reward
if not done:
target = (reward + self.gamma * np.amax(self.model.predict(next_state)[0]))
target_f = self.model.predict(state)
target_f[0][action] = target
self.model.fit(state, target_f, epochs=1, verbose=0)
if self.epsilon > self.epsilon_min:
self.epsilon *= self.epsilon_decay
```
5. 训练DQN代理
```python
env = gym.make('TankWar-ram-v0')
state_shape = env.observation_space.shape
action_shape = env.action_space.n
agent = DQNAgent(state_shape, action_shape)
batch_size = 32
num_episodes = 1000
num_steps = 500
for e in range(num_episodes):
state = env.reset()
state = np.reshape(state, [1, state_shape[0]])
for step in range(num_steps):
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, state_shape[0]])
agent.remember(state, action, reward, next_state, done)
state = next_state
if done:
break
agent.replay(batch_size)
```
6. 测试DQN代理
```python
state = env.reset()
state = np.reshape(state, [1, state_shape[0]])
for step in range(num_steps):
env.render()
action = agent.act(state)
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, state_shape[0]])
state = next_state
if done:
break
env.close()
```
这就是使用DQN实现坦克大战的完整代码。您可以使用以上代码来训练和测试您自己的DQN代理。
阅读全文
相关推荐

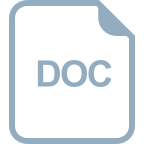



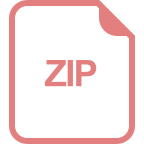
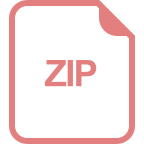








