输入一个中缀表达式,利用栈结构求解表达式的值。其中运算符包括:+、-、*、/、(、),表达式以“=”为结尾,参与运算的数据为double类型且为正数。
时间: 2023-04-29 17:06:40 浏览: 110
这段字符串是一个十六进制编码的字节串,需要解码成可读的字符。解码后的文字是:输入一个中缀表达式,利用栈结构求解表达式的值。其中运算符包括:+、-、*、/、(、),表达式以“=”为结尾,参与运算的数据为double类型且为正数。
相关问题
输入一个中缀算术表达式,求解表达式的值。运算符包括+、-、*、/、(、)、=,
好的,我可以帮您解析一个中缀算术表达式并计算其结果。首先需要将中缀表达式转换为后缀表达式,然后利用栈的特性进行计算。
具体步骤如下:
1. 创建一个栈和一个空列表作为结果集。
2. 遍历表达式中的每一个字符。
3. 如果字符是数字,将其添加到结果列表中。
4. 如果字符是左括号'(',将其压入栈中。
5. 如果字符是右括号')',则弹出栈中的所有运算符,直到遇到左括号为止,并将运算符添加到结果列表中。
6. 如果字符是运算符,将该运算符与栈顶运算符比较:
a. 如果栈为空,将该运算符压入栈中。
b. 如果该运算符优先级大于栈顶运算符,将其压入栈中。
c. 如果该运算符优先级小于或等于栈顶运算符,将栈顶运算符弹出并添加到结果列表中,重复步骤6直到满足条件b。
7. 如果遍历完了表达式,而栈中还有运算符,将其弹出并添加到结果列表中。
8. 根据后缀表达式,依次弹出运算数并进行计算,最终得到表达式的值。
下面是一个Python实现:
```python
# 定义运算符优先级
priority = {'+':1, '-':1, '*':2, '/':2, '(':0, ')':0}
# 将中缀表达式转换为后缀表达式
def infix_to_postfix(expression):
result = [] # 结果列表
stack = [] # 运算符栈
# 遍历表达式
for char in expression:
# 如果是数字,添加到结果列表
if char.isdigit():
result.append(char)
# 如果是左括号,压入栈中
elif char == '(':
stack.append(char)
# 如果是右括号,弹出栈中的运算符,并将其添加到结果列表中,直到遇到左括号
elif char == ')':
while stack and stack[-1] != '(':
result.append(stack.pop())
stack.pop() # 弹出左括号
# 如果是运算符,比较其与栈顶运算符的优先级
elif char in ['+', '-', '*', '/']:
while stack and priority[char] <= priority[stack[-1]]:
result.append(stack.pop())
stack.append(char)
else:
pass # 忽略=号
# 遍历结束后,弹出栈中剩余的运算符
while stack:
result.append(stack.pop())
return result
# 根据后缀表达式计算表达式的值
def calculate(postfix_expression):
stack = [] # 运算数栈
# 遍历后缀表达式
for char in postfix_expression:
if char.isdigit():
stack.append(int(char))
else:
b = stack.pop()
a = stack.pop()
if char == '+':
result = a + b
elif char == '-':
result = a - b
elif char == '*':
result = a * b
elif char == '/':
result = a / b
stack.append(result)
return stack[0]
# 测试
expression = input("请输入中缀表达式:")
postfix_expression = infix_to_postfix(expression)
result = calculate(postfix_expression)
print("表达式的值为:", result)
```
例如,输入表达式"3 * (1 + 4) / 2 = ",程序会输出"表达式的值为: 7.5"。
输入一个中缀算术表达式,求解表达式的值。运算符包括+、-、*、/、(、)、=,使用c++编写代码
我可以为您提供一个简单的中缀表达式求解程序,以下是使用C语言编写的代码:
```
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 50
// 符号栈结构体
struct stack {
int items[STACK_SIZE];
int top;
};
// 初始化符号栈
void init_stack(struct stack *s) {
s->top = -1;
}
// 判断符号栈是否为空
int is_empty(struct stack *s) {
return s->top == -1;
}
// 判断符号栈是否已满
int is_full(struct stack *s) {
return s->top == STACK_SIZE - 1;
}
// 入栈操作
void push(struct stack *s, int item) {
if (is_full(s)) {
printf("\nStack is full!");
exit(1);
}
s->items[++s->top] = item;
}
// 出栈操作
int pop(struct stack *s) {
if (is_empty(s)) {
printf("\nInvalid expression!");
exit(1);
}
return s->items[s->top--];
}
// 获取栈顶元素
int peek(struct stack *s) {
if (is_empty(s)) {
printf("\nInvalid expression!");
exit(1);
}
return s->items[s->top];
}
// 判断是否为操作符
int is_operator(char c) {
return (c == '+' || c == '-' || c == '*' || c == '/' || c == '(' || c == ')');
}
// 判断操作符的优先级
int precedence(char c) {
if (c == '(' || c == ')') {
return 0;
}
if (c == '+' || c == '-') {
return 1;
}
if (c == '*' || c == '/') {
return 2;
}
return 0;
}
// 运算操作
int operate(int op1, int op2, char operator) {
switch (operator) {
case '+':
return op1 + op2;
case '-':
return op1 - op2;
case '*':
return op1 * op2;
case '/':
return op1 / op2;
}
return 0;
}
// 解析中缀表达式
int evaluate(char *expression) {
struct stack operand_stack, operator_stack;
int i, n, operand1, operand2;
char token, operator;
init_stack(&operand_stack);
init_stack(&operator_stack);
n = strlen(expression);
for (i = 0; i < n; i++) {
token = expression[i];
// 如果是数字,压入操作数栈
if (isdigit(token)) {
push(&operand_stack, token - '0');
}
// 如果是左括号,压入操作符栈
else if (token == '(') {
push(&operator_stack, token);
}
// 如果是右括号,将操作符栈中左括号之间的操作符依次弹出,并进行运算,最后将左括号弹出
else if (token == ')') {
while (peek(&operator_stack) != '(') {
operand2 = pop(&operand_stack);
operand1 = pop(&operand_stack);
operator = pop(&operator_stack);
push(&operand_stack, operate(operand1, operand2, operator));
}
pop(&operator_stack);
}
// 如果是操作符,需要进行进一步判断
else if (is_operator(token)) {
// 如果当前操作符优先级小于等于操作符栈顶的操作符,将操作符栈顶的操作符依次弹出,并进行运算
while (!is_empty(&operator_stack) && precedence(token) <= precedence(peek(&operator_stack))) {
operand2 = pop(&operand_stack);
operand1 = pop(&operand_stack);
operator = pop(&operator_stack);
push(&operand_stack, operate(operand1, operand2, operator));
}
// 将当前操作符压入操作符栈
push(&operator_stack, token);
}
}
// 处理剩余的操作符
while (!is_empty(&operator_stack)) {
operand2 = pop(&operand_stack);
operand1 = pop(&operand_stack);
operator = pop(&operator_stack);
push(&operand_stack, operate(operand1, operand2, operator));
}
// 返回最终结果
return pop(&operand_stack);
}
int main() {
char expression[50];
int result;
printf("Please input an infix arithmetic expression:\n");
scanf("%s", expression);
result = evaluate(expression);
printf("\nResult = %d", result);
return 0;
}
```
使用该程序求解中缀表达式的值时,需要注意输入表达式时需用括号括起来,例如:(1+2)*3。
阅读全文
相关推荐












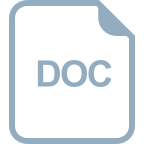

