本关任务:输入一个中缀算术表达式,求解表达式的值。运算符包括+、-、*、/、(、)、=,参加运算的数为 double 类型且为正数。(要求:直接针对中缀算术表达式进行计算,不能转换为后缀或前缀表达式再进行计算,只考虑二元运算即可。)
时间: 2024-12-28 10:37:29 浏览: 2
要解决这个任务,你需要设计一个程序来解析中缀算术表达式并计算其结果。这里的关键步骤包括:
1. **输入处理**:从用户那里获取输入字符串,确保它是有效的中缀表达式(即包含正确的运算符和数字,以及适当的括号结构)。
2. **符号栈**:使用栈数据结构来存储运算符。当遇到左括号时入栈,遇到右括号时弹出栈顶运算符直至遇到左括号为止,进行相应的运算。
3. **扫描与操作**:遍历输入字符串,对于数字字符,存储起来;对于运算符,执行以下操作:
- 如果遇到空格或换行,忽略。
- 如果遇到左括号,不进行任何操作。
- 如果遇到乘除法(* 和 /),寻找最近的两个操作数进行运算,并将结果压回栈。
- 如果遇到加减法(+ 和 -),寻找最近的未被覆盖的操作数进行运算,并将结果压回栈。
- 遇到等于号 (=),表示下一个操作数已经确定,不需要立即运算。
4. **计算结果**:在遍历完输入表达式后,栈中剩下的唯一元素就是最终的结果,因为它代表了没有与其他项相加减的最后一个操作数。
5. **特殊处理**:确保处理了所有可能的边界情况,比如空输入、只有一个操作数的情况等。
以下是一个简单的伪代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
double num;
char op;
} Token;
double evaluateExpression(char *input);
Token nextToken(char *input);
int main() {
char input[100];
printf("Enter an arithmetic expression (e.g., 2 + 3 * 4): ");
fgets(input, sizeof(input), stdin);
// Clean up newline character if present
input[strlen(input)-1] = '\0';
double result = evaluateExpression(input);
printf("Result: %.2f\n", result);
return 0;
}
// Evaluate the expression using a stack
double evaluateExpression(char *input) {
Token token;
Stack tokens = initStack();
// Scanning and parsing the expression
while (nextToken(input)) {
if (token.op == '+') {
double b = pop(tokens);
double a = pop(tokens);
push(tokens, {a + b});
} else if (token.op == '-') {
double b = pop(tokens);
double a = pop(tokens);
push(tokens, {a - b});
} else if (token.op == '*') {
double b = pop(tokens);
double a = pop(tokens);
push(tokens, {a * b});
} else if (token.op == '/') {
double b = pop(tokens);
double a = pop(tokens);
push(tokens, {a / b});
} else if (token.op == '=') {
// Invalid syntax; throw error or ignore it
} else if (isdigit(token.num)) {
push(tokens, token); // Keep numbers in the stack until they are used for operations
}
}
// Return the final result from the top of the stack
return pop(tokens).num;
}
// Helper function to get the next token from the input
Token nextToken(char *input) {
// Implement this function to extract the next token from the input string
}
```
请注意,上述代码只是一个基本框架,实际实现中你需要完善`nextToken()`函数来处理输入字符串中的每个字符,并正确处理边界情况。此外,根据需求,你还可以添加错误检查和提示功能,例如对于非法字符、缺少运算数等情况。
阅读全文
相关推荐
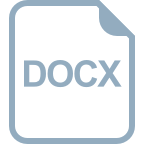
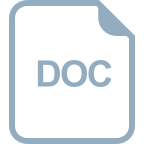
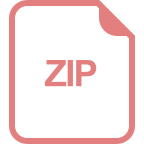
















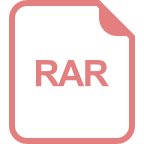