如何使用C++编程计算阶乘n!,即1*2*3*4*...*n,同时避免整数溢出问题?
时间: 2024-06-25 16:00:27 浏览: 7
在C++中,计算阶乘是一个常见的数学运算,特别是当n变得较大时,可能会遇到整数溢出的问题。为了避免溢出,可以使用迭代或递归的方式,并使用`long long`或者`__int128`这种高精度数据类型。这里提供两种方法:
**方法一:迭代计算**
```cpp
#include <iostream>
using namespace std;
// 使用长整型计算阶乘
long long factorial(int n) {
long long result = 1;
for (int i = 1; i <= n; ++i) {
result *= i;
// 如果result超过long long的最大值,就不再乘下去
if (result < 0) {
throw overflow_error("Factorial overflow");
}
}
return result;
}
int main() {
int n;
cout << "Enter a number: ";
cin >> n;
try {
long long fact = factorial(n);
cout << "Factorial of " << n << " is: " << fact << endl;
} catch (const overflow_error& e) {
cout << "Overflow occurred: " << e.what() << endl;
}
return 0;
}
```
**方法二:使用`std::numeric_limits`检查溢出**
```cpp
#include <iostream>
#include <limits>
using namespace std;
// 递归计算阶乘
long long factorial(int n) {
if (n < 0) {
throw invalid_argument("Negative numbers have no factorial");
} else if (n == 0 || n == 1) {
return 1;
} else {
// 在递归调用前检查可能的溢出
const long long max_value = numeric_limits<long long>::max();
if (n * factorial(n - 1) > max_value) {
throw overflow_error("Factorial overflow");
}
return n * factorial(n - 1);
}
}
int main() {
int n;
cout << "Enter a number: ";
cin >> n;
try {
long long fact = factorial(n);
cout << "Factorial of " << n << " is: " << fact << endl;
} catch (const overflow_error& e) {
cout << "Overflow occurred: " << e.what() << endl;
} catch (const invalid_argument& e) {
cout << "Invalid argument: " << e.what() << endl;
}
return 0;
}
```
相关推荐
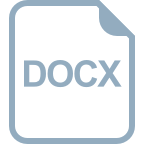
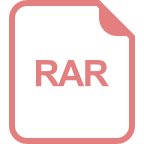
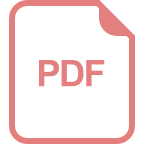














