写一个C语言的直方图增强代码
时间: 2023-08-31 07:36:12 浏览: 97
### 回答1:
以下是一个简单的C语言直方图增强代码的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PIXEL_VALUE 255
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s input_file output_file\n", argv[0]);
return 1;
}
FILE *input_file = fopen(argv[1], "rb");
if (!input_file) {
printf("Failed to open input file: %s\n", argv[1]);
return 1;
}
FILE *output_file = fopen(argv[2], "wb");
if (!output_file) {
printf("Failed to open output file: %s\n", argv[2]);
fclose(input_file);
return 1;
}
int histogram[MAX_PIXEL_VALUE + 1] = {0};
int pixel_count = 0;
// 计算直方图
int pixel;
while (fread(&pixel, sizeof(pixel), 1, input_file) == 1) {
histogram[pixel]++;
pixel_count++;
}
// 计算累积分布函数
int cdf[MAX_PIXEL_VALUE + 1] = {0};
cdf[0] = histogram[0];
for (int i = 1; i <= MAX_PIXEL_VALUE; i++) {
cdf[i] = cdf[i - 1] + histogram[i];
}
// 计算映射表
unsigned char map[MAX_PIXEL_VALUE + 1];
for (int i = 0; i <= MAX_PIXEL_VALUE; i++) {
map[i] = (unsigned char)(255.0 * cdf[i] / pixel_count + 0.5);
}
// 应用映射表
rewind(input_file);
while (fread(&pixel, sizeof(pixel), 1, input_file) == 1) {
unsigned char new_pixel = map[pixel];
fwrite(&new_pixel, sizeof(new_pixel), 1, output_file);
}
fclose(input_file);
fclose(output_file);
return 0;
}
```
这个程序接受两个命令行参数:输入文件和输出文件。它计算输入文件中像素值的直方图,然后计算累积分布函数和映射表,最后将输入文件中的每个像素值替换为映射表中对应的值,并将结果写入输出文件中。
### 回答2:
以下是一个用C语言实现的直方图增强代码作为示例:
```c
#include <stdio.h>
void histogramEqualization(int* image, int width, int height) {
int histogram[256] = {0}; // 建立直方图,初始化为0
int cumulativeHistogram[256] = {0}; // 累积直方图,初始化为0
int maxIntensity = 0;
// 计算每个像素的灰度直方图
for (int i = 0; i < width * height; i++) {
histogram[image[i]]++;
if (image[i] > maxIntensity) {
maxIntensity = image[i];
}
}
// 计算累积直方图
cumulativeHistogram[0] = histogram[0];
for (int i = 1; i <= maxIntensity; i++) {
cumulativeHistogram[i] = cumulativeHistogram[i - 1] + histogram[i];
}
// 进行直方图均衡化
for (int i = 0; i < width * height; i++) {
int enhancedIntensity = 255 * cumulativeHistogram[image[i]] / (width * height);
image[i] = enhancedIntensity;
}
}
int main() {
int image[8] = {10, 20, 30, 40, 50, 60, 70, 80};
int width = 2;
int height = 4;
printf("原始图像:\n");
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
printf("%3d ", image[i * width + j]);
}
printf("\n");
}
histogramEqualization(image, width, height);
printf("直方图增强后的图像:\n");
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
printf("%3d ", image[i * width + j]);
}
printf("\n");
}
return 0;
}
```
该代码实现了直方图增强算法。首先,通过计算输入图像的灰度直方图,获得每个灰度级别下的像素个数。然后,根据累积直方图公式,计算每个灰度级别的累计和。最后,根据累积直方图和图像的总像素数,计算每个像素在增强后的灰度级别。
在主函数中,我们定义了一个8个像素点的图像,并打印出原始图像。然后,调用histogramEqualization函数对图像进行直方图增强。最后,再次打印出增强后的图像。
注意:该示例代码仅为了说明直方图增强的基本原理,并未考虑实际应用中的一些优化和边界情况的处理。在实际应用中,可能需要对输入图像做预处理,如将彩色图像转为灰度图像;对直方图进行归一化处理等。
### 回答3:
下面是一个C语言的直方图增强代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
void histogram_equalization(int* image, int width, int height) {
int histogram[256] = {0}; // 初始化直方图数组,存储每个灰度级的像素个数
int cum_histogram[256] = {0}; // 累计直方图数组
// 计算直方图
for (int i = 0; i < width * height; i++) {
histogram[image[i]]++;
}
// 计算累计直方图
cum_histogram[0] = histogram[0];
for (int i = 1; i < 256; i++) {
cum_histogram[i] = cum_histogram[i - 1] + histogram[i];
}
// 直方图均衡化
int max_pixel = width * height;
for (int i = 0; i < width * height; i++) {
image[i] = (int)(((double)cum_histogram[image[i]] / max_pixel) * 255);
}
}
int main() {
int width = 3;
int height = 3;
int image[width * height] = { 1, 2, 3, 4, 5, 6, 7, 8, 9 };
histogram_equalization(image, width, height);
// 打印直方图均衡化后的图像
for (int i = 0; i < width * height; i++) {
printf("%d ", image[i]);
}
return 0;
}
```
这段代码实现了直方图增强的功能。首先,通过遍历图像像素计算出原始直方图。然后,根据原始直方图计算出累计直方图,用于后续的直方图均衡化操作。最后,遍历图像像素,将像素值映射到均衡化后的灰度级。在主函数中,可以输入自定义的图像大小和像素值,然后输出经过直方图增强后的图像像素值。
阅读全文
相关推荐










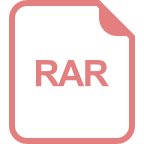







