输入AB值后,在C语言中使用辗转相除法,求出它们的最大公约数,再利用公式法求出最小公倍数的算法实现
时间: 2024-10-21 18:04:07 浏览: 30
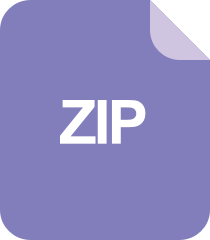
求最小公倍数的方法_C++_最小公倍数的几种求法_联合公倍数_

在C语言中,你可以使用欧几里得算法(辗转相除法)来计算两个数的最大公约数(Greatest Common Divisor, GCD),然后再根据最大公约数和乘积的关系来求出最小公倍数(Least Common Multiple, LCM)。以下是算法的实现步骤:
**辗转相除法(Euclidean Algorithm)求GCD:**
```c
#include <stdio.h>
// Function to calculate GCD using Euclid's algorithm
int gcd(int a, int b) {
if (b == 0)
return a; // base case: GCD of a and 0 is a
else
return gcd(b, a % b); // recursive call
}
int main() {
int num1, num2;
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
int result = gcd(num1, num2);
printf("The GCD of %d and %d is: %d\n", num1, num2, result);
// Now calculate LCM using formula: lcm(a, b) = |a*b| / gcd(a, b)
int lcm = abs(num1 * num2) / result;
printf("The LCM of %d and %d is: %d\n", num1, num2, lcm);
return 0;
}
```
在这个例子中,我们首先获取用户输入的两个数字,然后递归地调用`gcd`函数直到找到结果。之后,使用LCM的计算公式来求取最小公倍数。
阅读全文
相关推荐
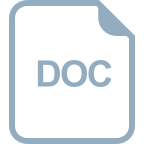
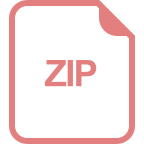
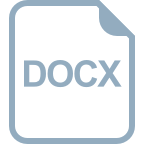
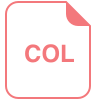
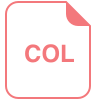
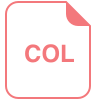






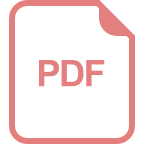
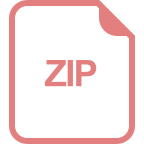
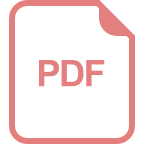