stm32f103c8t6四轮小车前进代码
时间: 2023-07-29 19:08:21 浏览: 112
以下是一个简单的 STM32F103C8T6 四轮小车前进的代码片段,使用 PWM 控制电机速度:
```c
#include "stm32f10x.h"
// 定义引脚和定时器
#define IN1_PIN GPIO_Pin_0
#define IN1_PORT GPIOA
#define IN2_PIN GPIO_Pin_1
#define IN2_PORT GPIOA
#define IN3_PIN GPIO_Pin_2
#define IN3_PORT GPIOA
#define IN4_PIN GPIO_Pin_3
#define IN4_PORT GPIOA
#define TIM_PERIOD 1000
void TIM3_Init(void);
void Motor_Init(void);
void Motor_Forward(void);
int main(void)
{
TIM3_Init();
Motor_Init();
while (1)
{
Motor_Forward();
}
}
void TIM3_Init(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// 使能定时器3和GPIOA时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置GPIO引脚为复用推挽输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 定时器3初始化
TIM_TimeBaseStructure.TIM_Prescaler = 7200 - 1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseStructure.TIM_Period = TIM_PERIOD - 1;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure);
// PWM初始化
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 0;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
// 配置通道1
TIM_OC1Init(TIM3, &TIM_OCInitStructure);
TIM_OC1PreloadConfig(TIM3, TIM_OCPreload_Enable);
// 配置通道2
TIM_OC2Init(TIM3, &TIM_OCInitStructure);
TIM_OC2PreloadConfig(TIM3, TIM_OCPreload_Enable);
// 使能定时器3
TIM_Cmd(TIM3, ENABLE);
}
void Motor_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 使能GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置引脚为推挽输出
GPIO_InitStructure.GPIO_Pin = IN1_PIN | IN2_PIN | IN3_PIN | IN4_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void Motor_Forward(void)
{
// 设置引脚电平,使电机前进
GPIO_SetBits(IN1_PORT, IN1_PIN);
GPIO_ResetBits(IN2_PORT, IN2_PIN);
GPIO_SetBits(IN3_PORT, IN3_PIN);
GPIO_ResetBits(IN4_PORT, IN4_PIN);
// 设置PWM占空比,控制电机速度
TIM_SetCompare1(TIM3, TIM_PERIOD / 2); // CH1 PWM占空比
TIM_SetCompare2(TIM3, TIM_PERIOD / 2); // CH2 PWM占空比
}
```
请注意,以上代码仅为示例,你需要根据你的具体硬件连接和需求进行适当的修改和配置。同时,你还需要在工程中添加正确的库文件和配置文件。希望对你有帮助!
阅读全文
相关推荐
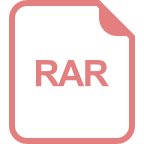
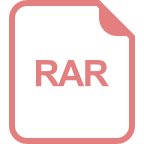
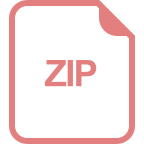
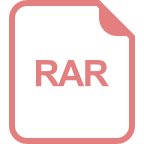










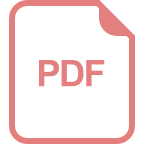
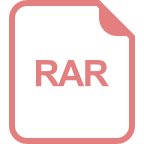
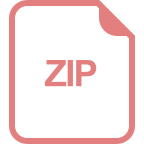