python pygame.time.Clock
时间: 2023-11-15 15:54:21 浏览: 177
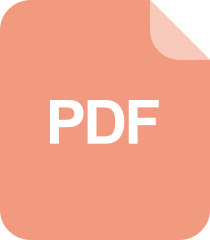
Python使用Pygame绘制时钟
`pygame.time.Clock` 是 Pygame 模块中的一个类,用于设置游戏循环的帧率。
在 Pygame 中,游戏循环是一个无限循环,用来处理游戏中的各种事件和更新游戏的状态。在每一次循环中,我们需要控制每秒钟的帧率,以避免游戏过度消耗 CPU 资源。
`pygame.time.Clock` 可以通过 `tick()` 方法控制每秒钟的帧率,并根据当前时间返回一个毫秒数,表示距离上一次调用 `tick()` 方法所经过的时间。
例如,下面的代码将每秒钟的帧率设置为 60 帧,并且在每次循环中打印经过的时间:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 创建屏幕
screen = pygame.display.set_mode((640, 480))
# 创建时钟
clock = pygame.time.Clock()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 退出游戏
pygame.quit()
sys.exit()
# 更新游戏状态
# 绘制屏幕
# 控制帧率
elapsed_time = clock.tick(60)
print(elapsed_time)
```
在上面的代码中,`clock.tick(60)` 控制了每秒钟的帧率,并返回距离上一次调用 `tick()` 方法所经过的时间。我们可以通过 `elapsed_time` 变量来获取这个时间,并在每次循环中打印出来。
阅读全文
相关推荐






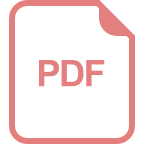
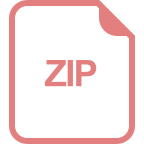
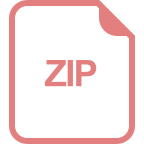
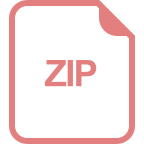
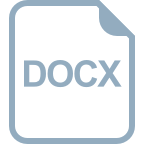




