Using pointers, write a function to swap the values of two ints. Test the function by calling it and printing the swapped values.
时间: 2024-05-07 22:22:05 浏览: 16
Here's an example implementation of a function that swaps the values of two integers using pointers:
```c++
#include <iostream>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int main() {
int x = 5, y = 10;
std::cout << "Before swap: x = " << x << ", y = " << y << std::endl;
swap(&x, &y);
std::cout << "After swap: x = " << x << ", y = " << y << std::endl;
return 0;
}
```
This program first defines the `swap` function, which takes two integer pointers as arguments and swaps the values they point to. It then defines two `int` variables `x` and `y`, sets them to 5 and 10 respectively, and prints their values. The `swap` function is then called with the address of `x` and `y` as arguments, causing their values to be swapped. Finally, the new values of `x` and `y` are printed again to confirm that the swap was successful.
相关推荐
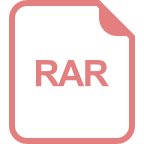
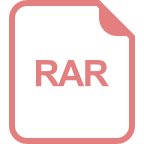















