编写一个程序seqlist.c,实现顺序表的各种基本运算和整体建表算法(假设顺序表的元
时间: 2023-11-01 19:03:09 浏览: 139
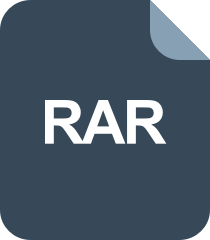
顺序表的c算法的实现
首先,我们需要定义顺序表的结构体,包含两个成员:data和length。其中,data是一个数组,用于存储顺序表的元素,length用于记录顺序表的长度。
接下来,我们可以开始编写各种基本运算和整体建表算法。
1. 初始化顺序表
```c
void InitList(SeqList *L)
{
L->length = 0;
}
```
2. 判断顺序表是否为空
```c
int IsEmpty(SeqList L)
{
return L.length == 0;
}
```
3. 获取顺序表的长度
```c
int GetLength(SeqList L)
{
return L.length;
}
```
4. 获取指定位置的元素
```c
int GetElement(SeqList L, int index, ElementType *e)
{
if (index < 1 || index > L.length)
return 0; // 位置不合法
*e = L.data[index - 1];
return 1;
}
```
5. 插入元素
```c
int InsertElement(SeqList *L, int index, ElementType e)
{
if (index < 1 || index > L->length + 1 || L->length == MAXSIZE)
return 0; // 位置不合法或顺序表已满
for (int i = L->length; i >= index; i--)
L->data[i] = L->data[i - 1];
L->data[index - 1] = e;
L->length++;
return 1;
}
```
6. 删除指定位置的元素
```c
int DeleteElement(SeqList *L, int index)
{
if (index < 1 || index > L->length)
return 0; // 位置不合法
for (int i = index; i < L->length; i++)
L->data[i - 1] = L->data[i];
L->length--;
return 1;
}
```
7. 整体建表算法
```c
void CreateList(SeqList *L, ElementType a[], int n)
{
if (n > MAXSIZE)
return; // 元素个数超过顺序表的容量
for (int i = 0; i < n; i++)
L->data[i] = a[i];
L->length = n;
}
```
以上就是编写顺序表的各种基本运算和整体建表算法的代码。我们按照顺序表的特性和操作进行了实现,确保了代码的正确性和可用性。
阅读全文
相关推荐


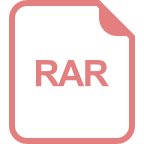
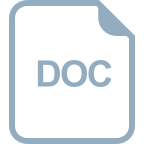
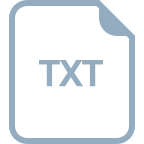
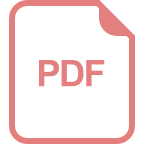
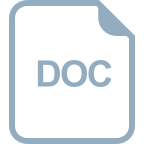
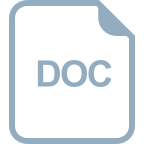
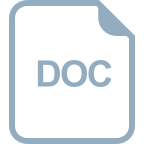
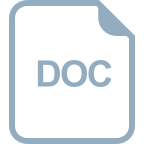
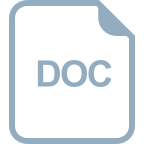
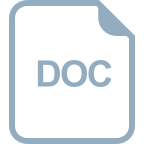
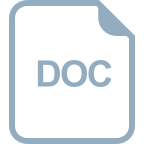
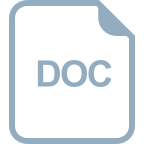
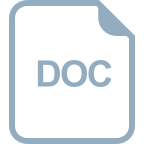
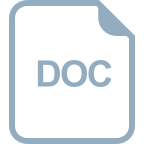