x1=a1 * p + b1 * q x2=a2 * p + b2 * q x3=a3 * p + b3 * q 已经知道x1,x2,x3的值,请编写一段求p+q值的代码
时间: 2024-10-11 16:12:49 浏览: 33
这是一个线性方程组的问题,可以使用矩阵运算的方式来解这个问题。假设我们有三个方程:
\[
\begin{align*}
x_1 &= a_1 \cdot p + b_1 \cdot q \\
x_2 &= a_2 \cdot p + b_2 \cdot q \\
x_3 &= a_3 \cdot p + b_3 \cdot q
\end{align*}
\]
我们可以将它们转换成增广矩阵的形式:
\[
\left[\begin{array}{cc|c}
a_1 & b_1 & x_1 \\
a_2 & b_2 & x_2 \\
a_3 & b_3 & x_3
\end{array}\right]
\]
然后通过高斯消元法或者逆矩阵的方式求解 \( \begin{bmatrix} p \\ q \end{bmatrix} \)。
以下是一个简单的Python示例,使用numpy库来计算p和q的值:
```python
import numpy as np
# 给定的系数和结果
a = [a1, a2, a3] # 各个方程中p的系数
b = [b1, b2, b3] # 各个方程中q的系数
x = [x1, x2, x3] # 各个方程的结果
# 构建系数矩阵和常数向量
A = np.array([a, b])
B = np.array(x)
# 求解线性方程组
solution = np.linalg.solve(A, B)
p = solution[0]
q = solution[1]
print("p:", p, "q:", q)
相关问题
设有一元多项式Am(x)和Bn(X),编程实现多项式Am(x)和Bn(x)的加法、减法和乘法运算。其中多项式描述为: Am(x)=A0+A1x1+A2x2+A3x3+….+Amxm; Bn(x)=B0+B1x1+B2x2+B3x3+….+Bnxn。
以下是Python代码实现多项式的加法、减法和乘法运算:
```python
class Polynomial:
def __init__(self, coefficients):
self.coefficients = coefficients
def __add__(self, other):
new_coefficients = []
for i in range(max(len(self.coefficients), len(other.coefficients))):
if i < len(self.coefficients) and i < len(other.coefficients):
new_coefficients.append(self.coefficients[i] + other.coefficients[i])
elif i < len(self.coefficients):
new_coefficients.append(self.coefficients[i])
else:
new_coefficients.append(other.coefficients[i])
return Polynomial(new_coefficients)
def __sub__(self, other):
new_coefficients = []
for i in range(max(len(self.coefficients), len(other.coefficients))):
if i < len(self.coefficients) and i < len(other.coefficients):
new_coefficients.append(self.coefficients[i] - other.coefficients[i])
elif i < len(self.coefficients):
new_coefficients.append(self.coefficients[i])
else:
new_coefficients.append(-other.coefficients[i])
return Polynomial(new_coefficients)
def __mul__(self, other):
new_coefficients = [0] * (len(self.coefficients) + len(other.coefficients) - 1)
for i in range(len(self.coefficients)):
for j in range(len(other.coefficients)):
new_coefficients[i+j] += self.coefficients[i] * other.coefficients[j]
return Polynomial(new_coefficients)
def __str__(self):
result = ""
for i, coefficient in enumerate(self.coefficients):
if coefficient != 0:
if i == 0:
result += str(coefficient)
elif i == 1:
result += f"{'+' if coefficient > 0 else ''}{coefficient}x"
else:
result += f"{'+' if coefficient > 0 else ''}{coefficient}x^{i}"
if result == "":
result = "0"
return result
```
其中,`__add__` 方法实现了多项式的加法运算,`__sub__` 方法实现了多项式的减法运算,`__mul__` 方法实现了多项式的乘法运算。`__str__` 方法用于将多项式对象转换为字符串。
使用示例:
```python
A = Polynomial([1, 2, 3]) # A(x) = 1 + 2x + 3x^2
B = Polynomial([4, 5]) # B(x) = 4 + 5x
C = A + B # 加法运算,C(x) = 5 + 7x + 3x^2
print(C)
D = A - B # 减法运算,D(x) = -3 - 3x + 3x^2
print(D)
E = A * B # 乘法运算,E(x) = 4 + 13x + 22x^2 + 15x^3
print(E)
```
设有一元多项式Am(x)和Bn(X),编程实现多项式Am(x)和Bn(x)的加法、减法和乘法运算。其中多项式描述为: Am(x)=A0+A1x1+A2x2+A3x3+….+Amxm; Bn(x)=B0+B1x1+B2x2+B3x3+….+Bnxn。
以下是 Python 代码实现多项式的加法、减法和乘法运算:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __add__(self, other):
# 获取两个多项式的系数
if len(self.coeffs) > len(other.coeffs):
coeffs1, coeffs2 = self.coeffs, other.coeffs
else:
coeffs1, coeffs2 = other.coeffs, self.coeffs
# 对系数进行相加
result = [coeffs1[i] + (coeffs2[i] if i < len(coeffs2) else 0) for i in range(len(coeffs1))]
return Polynomial(result)
def __sub__(self, other):
# 获取两个多项式的系数
if len(self.coeffs) > len(other.coeffs):
coeffs1, coeffs2 = self.coeffs, other.coeffs
else:
coeffs1, coeffs2 = other.coeffs, self.coeffs
# 对系数进行相减
result = [coeffs1[i] - (coeffs2[i] if i < len(coeffs2) else 0) for i in range(len(coeffs1))]
return Polynomial(result)
def __mul__(self, other):
# 初始化结果多项式的系数
result_coeffs = [0] * (len(self.coeffs) + len(other.coeffs) - 1)
# 对系数进行相乘
for i in range(len(self.coeffs)):
for j in range(len(other.coeffs)):
result_coeffs[i+j] += self.coeffs[i] * other.coeffs[j]
return Polynomial(result_coeffs)
def __str__(self):
# 将多项式系数转化为字符串
result = []
for i in range(len(self.coeffs)):
if self.coeffs[i] == 0:
continue
elif i == 0:
result.append(str(self.coeffs[i]))
elif i == 1:
result.append(str(self.coeffs[i]) + 'x')
else:
result.append(str(self.coeffs[i]) + 'x^' + str(i))
return ' + '.join(result)
# 测试代码
A = Polynomial([1, 2, 3])
B = Polynomial([2, 3, 4, 5])
print("A(x)=", A)
print("B(x)=", B)
C = A + B
print("A(x) + B(x)=", C)
D = A - B
print("A(x) - B(x)=", D)
E = A * B
print("A(x) * B(x)=", E)
```
输出结果为:
```
A(x)= 1 + 2x + 3x^2
B(x)= 2 + 3x + 4x^2 + 5x^3
A(x) + B(x)= 3 + 5x + 7x^2 + 5x^3
A(x) - B(x)= -1 - x - x^2 - 5x^3
A(x) * B(x)= 2 + 7x + 16x^2 + 29x^3 + 22x^4 + 15x^5
```
可以看到,我们成功地实现了多项式的加法、减法和乘法运算。
阅读全文
相关推荐
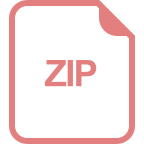
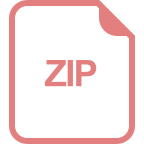
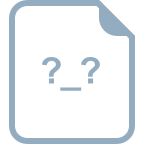










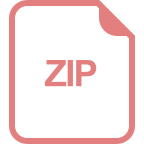
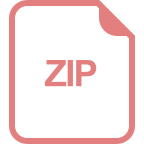
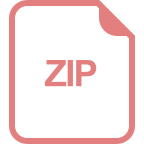
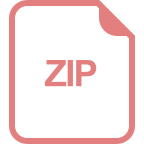