使用【python】写用迭代法求平方根的代码
时间: 2024-10-24 10:01:43 浏览: 62
在Python中,可以使用迭代法(如牛顿迭代法)来逼近一个数的平方根。这里是一个简单的例子,展示了如何使用迭代来计算一个正实数的平方根:
```python
def sqrt_iterative(number, epsilon=1e-6):
guess = number
while True:
new_guess = (guess + number / guess) / 2
if abs(new_guess - guess) < epsilon:
return round(new_guess, 6) # 返回近似值,保留小数点后六位
guess = new_guess
# 示例
number_to_square_root = 40
approximate_square_root = sqrt_iterative(number_to_square_root)
print(f"Approximate square root of {number_to_square_root} is {approximate_square_root}")
相关问题
python中如何使用迭代法求平方根
可以使用牛顿迭代法求平方根。算法如下:
1. 初始化一个猜测值 x0,比如 x0 = 1。
2. 通过以下公式计算下一个猜测值 x1:
x1 = (x0 + a/x0) / 2,其中 a 是要求平方根的数。
3. 判断新的猜测值 x1 是否已经足够接近真实平方根。如果是,则输出 x1;否则,将 x1 设为新的 x0,重复第 2 步。
下面是使用 Python 实现牛顿迭代法求平方根的代码:
```python
def sqrt(a):
x0 = 1.0
while True:
x1 = (x0 + a / x0) / 2
if abs(x1 - x0) < 1e-6:
return x1
x0 = x1
print(sqrt(2)) # 输出 1.4142135623746899
```
在这个例子中,我们求的是 2 的平方根。`1e-6` 表示 $10^{-6}$,即 0.000001,用于判断新的猜测值是否已经足够接近真实平方根。
python用牛顿迭代法求平方根_如何用牛顿法求一个数的平方根
牛顿迭代法是一种求解方程的数值方法,可以用来求一个数的平方根。对于求解方程 $f(x)=0$,牛顿迭代法的基本思想是:从初始估计值 $x_0$ 开始,通过不断迭代求解 $x_{n+1}=x_n-\frac{f(x_n)}{f'(x_n)}$,直到满足精度要求或迭代次数达到预设值为止。
对于求一个数 $a$ 的平方根,我们可以将其转化为求解 $f(x)=x^2-a=0$ 的根。根据牛顿迭代法的基本公式,可以得到求解平方根的迭代公式为:
$x_{n+1}=\frac{1}{2}(x_n+\frac{a}{x_n})$
其中,$x_0$ 可以取任意正实数,并且随着迭代次数的增加,$x_n$ 会越来越接近 $a$ 的平方根。
下面是 Python 代码示例:
```
def sqrt_newton(a, epsilon=1e-6):
x = a
while abs(x * x - a) > epsilon:
x = 0.5 * (x + a / x)
return x
```
其中,`a` 表示要求平方根的数,`epsilon` 表示迭代的精度要求,函数返回求得的平方根 `x`。
阅读全文
相关推荐
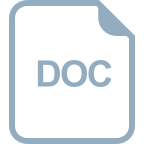
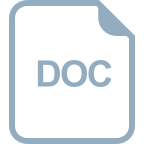
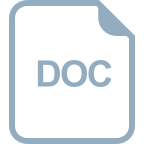
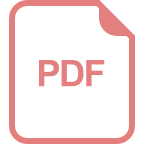







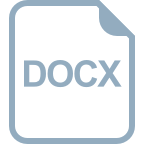




