5路巡线四轮小车环岛代码
时间: 2024-12-18 19:14:55 浏览: 5
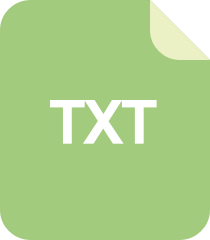
新建文本文档 (3).txt(四路红外巡线小车代码)
在编写一个5路巡线四轮小车环岛的代码时,通常涉及到使用PID控制(比例积分微分)算法来让车辆按照预定路径行驶。这里是一个简单的Python示例,使用了pygame库模拟一个基本的小车环岛控制:
```python
import pygame
import math
# 小车属性
class Robot:
def __init__(self, x, y):
self.x = x
self.y = y
self.speed = 5
self.rotation = 0
self.directions = [(1, 0), (0, 1), (-1, 0), (0, -1)] # 四个方向
# 游戏窗口设置
pygame.init()
screen = pygame.display.set_mode((800, 600))
robot = Robot(400, 300) # 初始化小车位置
def update():
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
robot.rotation -= 1
elif keys[pygame.K_RIGHT]:
robot.rotation += 1
else:
robot.rotation = 0
target_direction = rotate(robot.directions[robot.rotation % 4], robot.rotation)
robot.x += target_direction[0] * robot.speed
robot.y += target_direction[1] * robot.speed
# 环岛处理
if robot.x + robot.speed > screen.get_width() or robot.x < 0:
robot.x = 0 if robot.x + robot.speed > screen.get_width() else screen.get_width() - robot.speed
if robot.y + robot.speed > screen.get_height() or robot.y < 0:
robot.y = 0 if robot.y + robot.speed > screen.get_height() else screen.get_height() - robot.speed
def rotate(direction, rotation):
return [direction[0] * math.cos(math.radians(rotation)) - direction[1] * math.sin(math.radians(rotation)),
direction[0] * math.sin(math.radians(rotation)) + direction[1] * math.cos(math.radians(rotation))]
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
update()
screen.fill((255, 255, 255))
pygame.draw.rect(screen, (255, 0, 0), (robot.x, robot.y, 50, 50), 0)
pygame.display.flip()
```
这个代码创建了一个小车,通过用户按键改变方向,并在环形区域内保持循环。注意,这只是一个基础示例,实际应用可能需要更复杂的路径规划和障碍物检测。
阅读全文
相关推荐
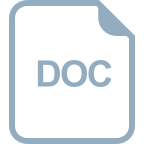
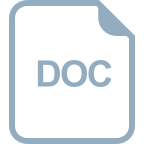
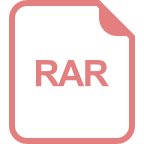






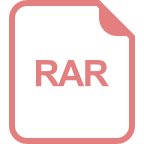






