定义一个函数,将输入的tensor格式图片加上不规则的掩膜,输出带有掩膜的tensor图像以及掩膜本身
时间: 2024-05-07 22:16:51 浏览: 258
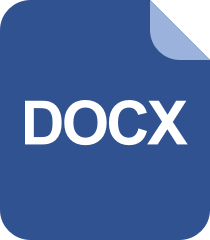
图像处理中的函数
好的,这是一个使用 PyTorch 的例子,定义一个函数来处理输入的图片和掩模:
```python
import torch
import numpy as np
def add_mask(image_tensor):
# 创建一个随机的掩模
mask = torch.zeros(image_tensor.shape[2], image_tensor.shape[3])
x = np.random.randint(0, image_tensor.shape[3] - 10)
y = np.random.randint(0, image_tensor.shape[2] - 10)
mask[x:x+10, y:y+10] = 1.0
# 创建一个新的 tensor 并将其与掩模相乘
masked_image = torch.zeros_like(image_tensor)
masked_image[:] = image_tensor[:]
for i in range(masked_image.shape[0]):
masked_image[i, :, :] *= mask
# 返回掩模和带有掩模的图像
return masked_image, mask
```
你可以将此函数应用于您的输入 tensor 图像,使用以下代码:
```python
import torch
import torchvision.transforms as transforms
from PIL import Image
# 读取输入 tensor 图片
image_path = "/path/to/image"
image = Image.open(image_path).convert("RGB")
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
])
image_tensor = transform(image).unsqueeze(0)
# 应用掩模
masked_image, mask = add_mask(image_tensor)
# 显示掩模和带有掩模的图像
import matplotlib.pyplot as plt
plt.imshow(mask.numpy(), cmap="gray")
plt.show()
masked_image = masked_image.squeeze(0).numpy().transpose((1, 2, 0))
plt.imshow(masked_image)
plt.show()
```
此代码将读取图像并将其转换为 PyTorch tensor 格式,然后应用掩模。最后,它将显示掩模和带有掩模的图像。
阅读全文
相关推荐
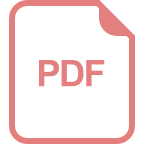
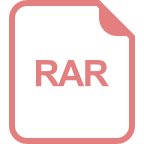















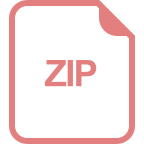