定义表示代数多项式中一项的结构体struct Item类型如下: double coeff;//多项式系数 int power;//x的幂 在主函数main()中定义struct Item类型变量item1,从键盘输入数据赋给item1的各成员,再输入x的值,计算该项值,并显示。 为结构体struct Item类型增加struct Item* next成员。并以此为结点,使用malloc函数动态建立多项式单链表。程序结束前使用free函数释放单链表所有结点。用c语言编写
时间: 2024-03-23 18:36:56 浏览: 15
好的,以下是 C 语言实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
struct Item {
double coeff;
int power;
struct Item* next;
};
double calculate(struct Item item, double x) {
return item.coeff * pow(x, item.power);
}
int main() {
struct Item item1;
printf("Enter coefficient: ");
scanf("%lf", &item1.coeff);
printf("Enter power: ");
scanf("%d", &item1.power);
printf("Enter x value: ");
double x;
scanf("%lf", &x);
printf("Value of item1: %lf\n", calculate(item1, x));
struct Item* head = NULL;
int num;
printf("Enter number of items in polynomial: ");
scanf("%d", &num);
for (int i = 0; i < num; i++) {
struct Item* newItem = (struct Item*)malloc(sizeof(struct Item)); // 使用 malloc 动态分配内存
printf("Enter coefficient of item %d: ", i+1);
scanf("%lf", &newItem->coeff);
printf("Enter power of item %d: ", i+1);
scanf("%d", &newItem->power);
newItem->next = head;
head = newItem;
}
double total = 0.0;
struct Item* current = head;
while (current != NULL) {
total += calculate(*current, x);
current = current->next;
}
printf("Value of polynomial: %lf\n", total);
current = head;
while (current != NULL) {
struct Item* temp = current;
current = current->next;
free(temp); // 使用 free 释放内存
}
return 0;
}
```
与 C++ 版本类似,这里也先定义了一个 `calculate` 函数,用于计算某一项在给定 `x` 值时的值。然后在 `main` 函数中先输入一个项并计算其值,然后动态建立多项式的单链表,输入每一项的系数和幂,同时将其作为一个新的结点插入链表头。最后遍历链表计算多项式的值,然后使用 `free` 释放链表中每个结点的内存。需要注意的是,这里使用了 `struct` 关键字来定义结构体类型。
相关推荐
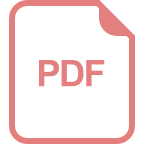
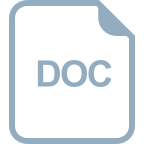
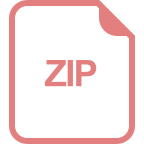












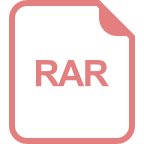
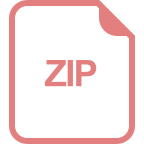
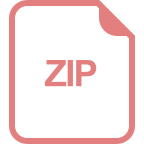