已知一个非纯集合B,试构造一个纯集合A,使A中只包含B中所有值 各不相同的数据元素。用类C语言写出算法,并计算时间复杂度。
时间: 2024-03-22 12:37:12 浏览: 65
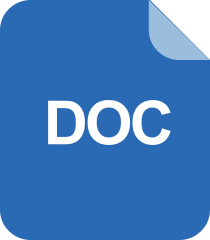
数据结构算法时间复杂度的计算.doc
可以使用哈希表来实现。
首先,遍历集合B中的每一个数据元素,将其插入到哈希表中。在插入前,先检查哈希表中是否已经存在该元素,若存在则不插入。这样可以保证纯集合A中只包含B中所有值各不相同的数据元素。
以下是用类C语言写出的算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_SIZE 100 // 假设集合B中最多有100个元素
// 哈希表中的节点结构体
typedef struct HashNode {
int key; // 键值,即数据元素
struct HashNode* next; // 下一个节点指针
} HashNode;
// 哈希表结构体
typedef struct HashTable {
HashNode** data; // 数组,存放指向节点的指针
int size; // 哈希表大小
} HashTable;
// 创建哈希表
HashTable* createHashTable(int size) {
HashTable* hashTable = (HashTable*)malloc(sizeof(HashTable));
hashTable->data = (HashNode**)malloc(sizeof(HashNode*) * size);
hashTable->size = size;
for (int i = 0; i < size; i++) {
hashTable->data[i] = NULL;
}
return hashTable;
}
// 计算哈希值
int hash(int key, int size) {
return key % size;
}
// 在哈希表中查找节点
HashNode* findNode(HashTable* hashTable, int key) {
int index = hash(key, hashTable->size);
HashNode* p = hashTable->data[index];
while (p != NULL) {
if (p->key == key) {
return p;
}
p = p->next;
}
return NULL;
}
// 在哈希表中插入节点
void insertNode(HashTable* hashTable, int key) {
HashNode* node = findNode(hashTable, key);
if (node == NULL) {
int index = hash(key, hashTable->size);
node = (HashNode*)malloc(sizeof(HashNode));
node->key = key;
node->next = hashTable->data[index];
hashTable->data[index] = node;
}
}
// 构造纯集合
void constructPureSet(int* B, int size) {
HashTable* hashTable = createHashTable(size);
for (int i = 0; i < size; i++) {
insertNode(hashTable, B[i]);
}
for (int i = 0; i < hashTable->size; i++) {
HashNode* p = hashTable->data[i];
while (p != NULL) {
printf("%d ", p->key);
p = p->next;
}
}
}
int main() {
int B[MAX_SIZE] = {1, 2, 3, 2, 4, 1, 5, 6, 3};
int size = 9;
constructPureSet(B, size);
return 0;
}
```
算法的时间复杂度为O(n),其中n为集合B的大小。
阅读全文
相关推荐


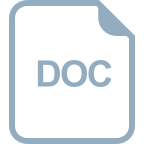
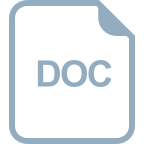











