java 连接opc ua的代码
时间: 2023-08-23 13:07:03 浏览: 66
下面是一个简单的 Java 代码示例,用于连接 OPC UA 服务器和读取其中的数据。这个代码示例使用 Eclipse Milo 库来实现 OPC UA 功能。
```java
import org.eclipse.milo.opcua.sdk.client.OpcUaClient;
import org.eclipse.milo.opcua.sdk.client.api.identity.UsernameProvider;
import org.eclipse.milo.opcua.sdk.client.model.nodes.objects.ServerNode;
import org.eclipse.milo.opcua.stack.core.types.builtin.DataValue;
import org.eclipse.milo.opcua.stack.core.types.builtin.NodeId;
import org.eclipse.milo.opcua.stack.core.types.builtin.Variant;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class OpcUaClientExample {
public static void main(String[] args) throws InterruptedException, ExecutionException {
// 创建 OPC UA 客户端
OpcUaClient client = OpcUaClient.create("opc.tcp://localhost:12686/UA/MyLittleServer");
// 设置用户凭证(如果需要)
client.setIdentityProvider(new UsernameProvider("user", "password".toCharArray()));
// 连接 OPC UA 服务器
CompletableFuture<OpcUaClient> future = client.connect();
future.get();
// 读取 OPC UA 服务器上的节点数据,例如服务器版本号
NodeId nodeId = new NodeId(2, "i=2261");
CompletableFuture<DataValue> readFuture = client.readValue(0, null, nodeId);
DataValue dataValue = readFuture.get();
Variant variant = dataValue.getValue();
Object value = variant.getValue();
System.out.println("Server version: " + value);
// 关闭 OPC UA 客户端
client.disconnect().get();
}
}
```
在上面的代码示例中,我们首先创建了一个 OPC UA 客户端对象,然后设置了 OPC UA 服务器的 URL 地址和用户凭证(如果需要)。接着,我们使用 `connect()` 方法连接 OPC UA 服务器,这个方法返回一个 `CompletableFuture<OpcUaClient>` 对象,我们需要使用 `get()` 方法等待连接成功。连接成功后,我们就可以使用客户端对象的各种方法来读取 OPC UA 服务器上的节点数据。在上面的代码示例中,我们读取了服务器版本号,并将其打印到控制台上。最后,我们使用 `disconnect()` 方法关闭了 OPC UA 客户端。
相关推荐
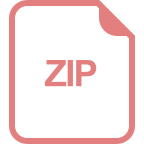
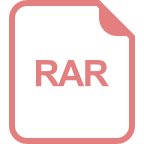














