写一段代码,在OpenGl上绘制一个图形,并实现基于种子点的区域填充算法,然后调用该算法对绘制图形指定区域进行填充,把这个图形涂成蓝色。
时间: 2024-05-18 21:15:06 浏览: 19
好的,这是一个使用OpenGL绘制一个多边形,并实现基于种子点的区域填充算法的代码示例。
```c++
#include <GL/glut.h>
#include <vector>
#include <stack>
using namespace std;
// 定义一个点的数据结构
struct Point {
int x, y;
};
// 定义一个多边形的数据结构
struct Polygon {
vector<Point> vertices;
};
// 定义一个用于区域填充的种子点
struct Seed {
int x, y;
};
// 定义一个变量表示填充颜色
GLfloat blue[] = { 0.0, 0.0, 1.0, 1.0 };
// 定义一个函数用于绘制多边形
void drawPolygon(Polygon polygon) {
glBegin(GL_LINE_LOOP);
for (int i = 0; i < polygon.vertices.size(); i++) {
glVertex2i(polygon.vertices[i].x, polygon.vertices[i].y);
}
glEnd();
}
// 定义一个函数用于填充区域
void floodFill(int x, int y) {
// 定义一个栈用于保存种子点
stack<Seed> seeds;
Seed seed = { x, y };
seeds.push(seed);
// 循环处理所有种子点
while (!seeds.empty()) {
Seed currentSeed = seeds.top();
seeds.pop();
// 获取当前种子点的颜色
GLfloat currentColor[4];
glReadPixels(currentSeed.x, currentSeed.y, 1, 1, GL_RGBA, GL_FLOAT, currentColor);
// 如果当前颜色不是蓝色,则填充当前点
if (currentColor[0] != blue[0] || currentColor[1] != blue[1] || currentColor[2] != blue[2]) {
glBegin(GL_POINTS);
glVertex2i(currentSeed.x, currentSeed.y);
glEnd();
// 将当前点上下左右的点作为新的种子点入栈
Seed left = { currentSeed.x - 1, currentSeed.y };
Seed right = { currentSeed.x + 1, currentSeed.y };
Seed up = { currentSeed.x, currentSeed.y + 1 };
Seed down = { currentSeed.x, currentSeed.y - 1 };
seeds.push(left);
seeds.push(right);
seeds.push(up);
seeds.push(down);
}
}
}
// 定义多边形和种子点
Polygon polygon = { {{100, 100}, {200, 100}, {150, 200}} };
Seed seed = { 150, 150 };
// 定义OpenGL显示函数
void display() {
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0, 1.0, 1.0);
drawPolygon(polygon);
glColor4fv(blue);
floodFill(seed.x, seed.y);
glFlush();
}
// 定义OpenGL初始化函数
void init() {
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, 400, 0, 400);
}
// 主函数
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(400, 400);
glutInitWindowPosition(100, 100);
glutCreateWindow("Flood Fill Algorithm");
init();
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
这个程序使用OpenGL库绘制一个三角形,并使用基于种子点的区域填充算法将其中一个区域涂成蓝色。在程序中,`drawPolygon()`函数用于绘制多边形,`floodFill()`函数用于填充区域,`display()`函数用于在OpenGL窗口中显示图形,`init()`函数用于初始化OpenGL环境,`main()`函数是主函数。
相关推荐
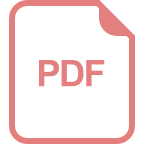














