通过栈来实现中缀表达式转后缀表达式
时间: 2024-01-06 15:22:22 浏览: 100
中缀表达式转后缀表达式的过程可以通过栈来实现。具体步骤如下:
1. 初始化两个栈,一个用于存储运算符的栈OPTR,一个用于存储转换后的后缀表达式的栈OPND。
2. 从左到右扫描中缀表达式,如果遇到操作数,则直接压入OPND栈中;如果遇到运算符,则与OPTR栈顶元素进行比较,如果该运算符优先级高于OPTR栈顶元素,则将该运算符压入OPTR栈中;否则,将OPTR栈顶元素弹出并压入OPND栈中,直到该运算符优先级高于OPTR栈顶元素或OPTR栈为空,然后将该运算符压入OPTR栈中。
3. 如果遇到左括号,则直接压入OPTR栈中;如果遇到右括号,则将OPTR栈顶元素弹出并压入OPND栈中,直到遇到左括号,然后将左括号弹出。
4. 当扫描完整个中缀表达式后,将OPTR栈中剩余的运算符依次弹出并压入OPND栈中。
5. 最后,将OPND栈中的元素依次弹出,即可得到转换后的后缀表达式。
下面是一个示例代码,供参考:
```
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int priority(char op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
string infixToPostfix(string infix) {
stack<char> optr;
string postfix = "";
for (int i = 0; i < infix.length(); i++) {
char ch = infix[i];
if (isdigit(ch)) {
postfix += ch;
} else if (ch == '(') {
optr.push(ch);
} else if (ch == ')') {
while (optr.top() != '(') {
postfix += optr.top();
optr.pop();
}
optr.pop();
} else {
while (!optr.empty() && priority(ch) <= priority(optr.top())) {
postfix += optr.top();
optr.pop();
}
optr.push(ch);
}
}
while (!optr.empty()) {
postfix += optr.top();
optr.pop();
}
return postfix;
}
int main() {
string infix = "1+(2-3)*4+10/5";
string postfix = infixToPostfix(infix);
cout << postfix << endl;
return 0;
}
```
阅读全文
相关推荐

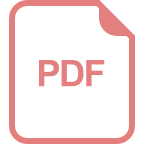
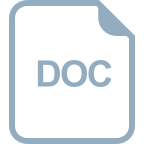









