c++编程序实现单链表,并且使用单链表实现多项式相加
时间: 2024-09-17 08:08:33 浏览: 70
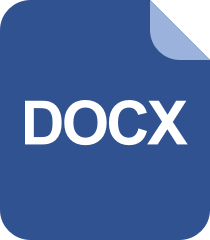
c++数据结构实验:多项式相加

在C++中,我们可以使用结构体(struct)和指针来实现单链表。首先,我们创建一个表示节点的结构体,包含数据域和指向下一个节点的指针:
```cpp
// 定义链表节点
struct ListNode {
int data; // 数据域
ListNode* next; // 指向下一个节点的指针
};
```
然后,我们可以定义一些基本操作,如初始化、添加元素到链表尾部以及遍历链表:
```cpp
// 初始化空链表
ListNode* createEmptyList() {
return nullptr;
}
// 添加元素到链表尾部
ListNode* addElement(ListNode*& head, int value) {
if (head == nullptr) {
head = new ListNode{value, nullptr};
} else {
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new ListNode{value, nullptr};
}
return head;
}
// 遍历并打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << std::endl;
}
```
接下来,为了实现多项式的相加,我们可以定义两个链表分别存储多项式的系数和指数。可以创建一个函数接收两个链表作为输入,然后逐项相加:
```cpp
// 函数用于计算两个多项式的和
ListNode* addPolynomials(ListNode* poly1_coefficient, ListNode* poly2_coefficient) {
ListNode* result_head = nullptr;
int carry = 0;
while (poly1_coefficient != nullptr || poly2_coefficient != nullptr) {
int sum = (poly1_coefficient ? poly1_coefficient->data : 0)
+ (poly2_coefficient ? poly2_coefficient->data : 0)
+ carry;
carry = sum / 10; // 计算进位
ListNode* newNode = new ListNode{sum % 10, nullptr}; // 新节点的数据是当前项的值
if (!result_head) {
result_head = newNode;
} else {
ListNode* current = result_head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
if (poly1_coefficient) {
poly1_coefficient = poly1_coefficient->next;
}
if (poly2_coefficient) {
poly2_coefficient = poly2_coefficient->next;
}
}
if (carry > 0) {
newNode = new ListNode{carry, nullptr};
if (result_head) {
ListNode* current = result_head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
} else {
result_head = newNode;
}
}
return result_head;
}
```
阅读全文
相关推荐
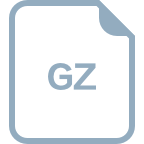
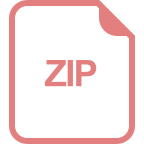
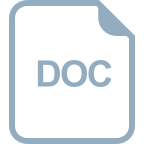
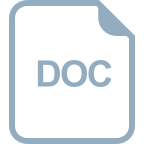
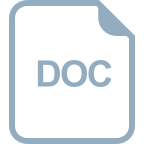



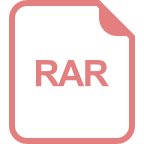
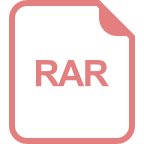
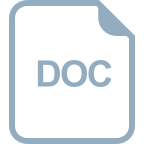





