设计整数单链表的基础运算程序
时间: 2024-10-17 20:15:50 浏览: 27
设计一个整数单链表基础运算是指创建链表结构,并实现常见的操作,如插入节点、删除节点、查找节点以及打印链表等。这里是一个简单的示例:
1. **定义链表节点**:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
```
2. **初始化空链表**:
```python
def create_empty_list():
return ListNode()
```
3. **插入节点**:
```python
def insert_node(head, val):
new_node = ListNode(val)
if not head:
head = new_node
else:
current = head
while current.next:
current = current.next
current.next = new_node
return head
```
4. **删除节点** (假设给定值存在于链表中):
```python
def delete_node(head, val):
if not head:
return None
if head.val == val:
return head.next
current = head
while current.next and current.next.val != val:
current = current.next
if current.next:
current.next = current.next.next
return head
```
5. **查找节点**:
```python
def find_node(head, val):
current = head
while current:
if current.val == val:
return True
current = current.next
return False
```
6. **打印链表**:
```python
def print_list(head):
current = head
while current:
print(current.val, end=" -> ")
current = current.next
print("None")
```
阅读全文
相关推荐
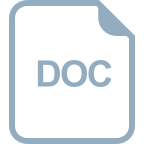
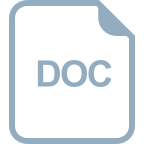
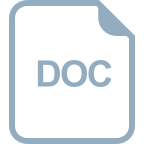














