梯度下降法python
时间: 2023-10-29 13:56:23 浏览: 36
梯度下降法是一种常用的优化算法,用于求解函数的最小值。在Python中,我们可以使用NumPy库来实现梯度下降法。
以下是一个基本的梯度下降法的Python示例代码:
```python
import numpy as np
def gradient_descent(X, y, learning_rate=0.01, num_iterations=1000):
num_samples, num_features = X.shape
theta = np.zeros(num_features)
cost_history = []
for i in range(num_iterations):
# 计算预测值
y_pred = np.dot(X, theta)
# 计算误差
error = y_pred - y
# 计算梯度
gradient = np.dot(X.T, error) / num_samples
# 更新参数
theta -= learning_rate * gradient
# 计算损失函数值
cost = np.sum(error ** 2) / (2 * num_samples)
cost_history.append(cost)
return theta, cost_history
```
在上述代码中,X是输入特征矩阵,y是目标变量向量。learning_rate表示学习率,控制参数更新的步长。num_iterations表示迭代次数。
你可以根据自己的数据和需求调整学习率和迭代次数。函数返回优化后的参数theta和每次迭代的损失函数值。
使用示例:
```python
import numpy as np
# 构造样本数据
X = np.array([[1, 1], [1, 2], [1, 3], [1, 4]])
y = np.array([2, 3, 4, 5])
# 调用梯度下降法函数
theta, cost_history = gradient_descent(X, y, learning_rate=0.01, num_iterations=1000)
# 输出结果
print("Optimized parameters:", theta)
print("Final cost:", cost_history[-1])
```
这是一个简单的梯度下降法的实现,你可以根据自己的需求进行修改和优化。希望对你有所帮助!如果有更多问题,可以继续问我。
相关推荐
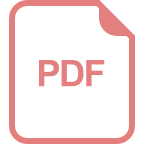
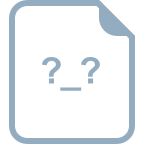













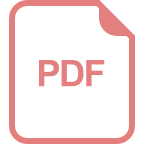