vs2019中基于PCL1.12.1的点云形状特征提取方式的C++
时间: 2024-10-08 21:24:43 浏览: 33
在Visual Studio 2019 (VS2019) 中,如果你想要基于Point Cloud Library (PCL) 1.12.1 版本对点云进行形状特征提取,PCL 提供了一套完整的工具集用于处理3D点云数据,包括形状分析。以下是使用 C++ 进行基本操作的一些建议:
1. **安装PCL**:首先确保已安装了PCL库及其依赖项。可以在官网下载源码包并按照文档进行编译安装。
2. **包含头文件**:在C++代码中,需要包含相关的PCL头文件,例如 `pcl/point_types.h`、`pcl/features/feature.h` 和 `pcl/features/normal_3d.h` 等,它们包含了形状特征提取所需的函数和结构体。
3. **加载点云数据**:使用 PCL 的 `pcl::PointCloud<pcl::PointXYZ>::Ptr` 或 `pcl::PointXYZI` 类型从文件或实时输入读取点云数据。
4. **预处理步骤**:对点云进行平滑、滤波等预处理操作,以便于后续的形状特征提取,可以使用 `pcl::PassThrough` 或 `pcl::VoxelGrid`。
5. **计算局部描述符**:对于形状描述,PCL提供了如FPFH (Fast Point Feature Histograms) 或 SHOT (Signature of Histogram Oriented Gradients) 等算法,通过 `pcl::FeatureFromNormals` 类实现。
6. **特征聚类**:如果想获取形状模式,可以使用 `pcl::MeanShift` 或其他聚类算法对特征点进行分组。
```cpp
#include <pcl/features/feature.h>
#include <pcl/point_types.h>
// ...
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
// ... 加载数据
pcl::NormalEstimation<pcl::PointXYZ, pcl:: Normal> ne;
ne.setInputCloud(cloud);
ne.setKSearch(30); // 设置搜索邻居数
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>);
ne.setSearchMethod(tree);
pcl::search::RadiusSearch<pcl::Normal> rs;
rs.setInputCloud(ne.getSearchSurface());
rs.setRadiusSearch(0.03); // 设置半径范围
pcl::FeatureFromNormals<pcl::PointXYZ, pcl::Normal> feature;
feature.setInputCloud(cloud);
feature.setInputNormals(rs.compute(*cloud));
feature.setRadiusSearch(rs);
feature.setHistogramSize(32); // 设置直方图大小
std::vector<pcl::FPFHSignature33> descriptors;
feature.compute(descriptors);
```
相关推荐
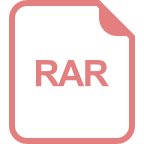
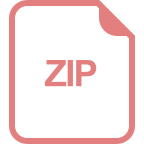
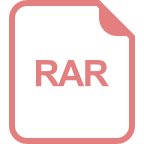














