用c语言编写一个程序,使用递归法方法计算n!
时间: 2024-10-24 19:08:18 浏览: 41
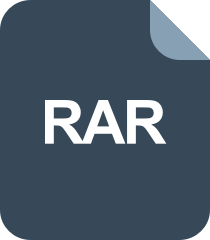
用C语言编写牛顿插值程序.rar_C语言_插值 c语言_牛顿插值_牛顿插值c
在C语言中,我们可以使用递归函数来计算阶乘(n!)。下面是一个简单的示例:
```c
#include <stdio.h>
// 定义阶乘函数,采用递归方式实现
unsigned long long factorial(int n) {
if (n == 0 || n == 1) { // 递归的基本情况,0! 和 1! 都等于1
return 1;
} else {
// 递归调用自身,n! = n * (n- 1);
}
}
int main() {
int num;
printf("请输入一个非负整数:");
scanf("%d", &num);
// 检查输入是否合法
if (num < 0) {
printf("错误:输入值必须是非负整数。\n");
} else {
unsigned long long result = factorial(num);
printf(" %d 的阶乘是:%llu\n", num, result);
}
return 0;
}
```
当你运行这个程序并输入一个正整数,它会计算并打印出该数字的阶乘。
阅读全文
相关推荐
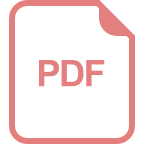
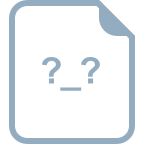















