LINUX下从串口接收指定长度的数据
时间: 2023-10-29 07:04:31 浏览: 110
在Linux下,你可以使用串口设备文件来接收指定长度的数据。以下是一个基本的示例代码,你可以根据自己的需求进行修改:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main() {
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY); // 打开串口设备文件,根据实际情况修改设备文件路径
if (fd == -1) {
perror("无法打开串口设备文件");
return -1;
}
struct termios config;
if (tcgetattr(fd, &config) < 0) {
perror("无法获取串口配置");
close(fd);
return -1;
}
// 设置串口波特率为9600
cfsetispeed(&config, B9600);
cfsetospeed(&config, B9600);
// 设置数据位为8位
config.c_cflag &= ~CSIZE;
config.c_cflag |= CS8;
// 设置无奇偶校验
config.c_cflag &= ~PARENB;
// 设置停止位为1位
config.c_cflag &= ~CSTOPB;
// 禁用硬件流控制
config.c_cflag &= ~CRTSCTS;
// 设置为原始模式
config.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
// 设置读取最少字符数和等待时间
config.c_cc[VMIN] = 1;
config.c_cc[VTIME] = 10; // 读取超时时间为1秒
// 应用配置
if (tcsetattr(fd, TCSAFLUSH, &config) < 0) {
perror("无法应用串口配置");
close(fd);
return -1;
}
char buffer[100]; // 接收数据的缓冲区
ssize_t count = read(fd, buffer, sizeof(buffer)); // 从串口读取数据
if (count == -1) {
perror("无法读取数据");
close(fd);
return -1;
}
printf("接收到的数据: %.*s\n", (int)count, buffer);
close(fd);
return 0;
}
```
上述代码打开了 `/dev/ttyS0` 设备文件,设置了波特率为 9600,数据位为 8 位,无奇偶校验,停止位为 1 位,禁用了硬件流控制,并设置了读取最少字符数为 1,超时时间为 1 秒。然后通过 `read` 函数从串口读取数据,并将读取到的数据打印出来。
你可以根据实际需求修改代码中的设备文件路径、波特率等参数。注意,在运行代码前,你需要有相应的权限来访问串口设备文件(如 `/dev/ttyS0`)。
希望这能帮到你!如果有任何问题,请随时提问。
阅读全文
相关推荐
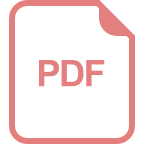
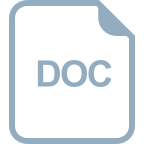




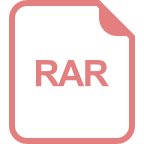
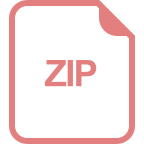
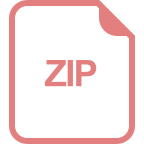
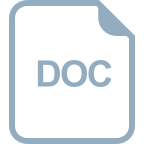
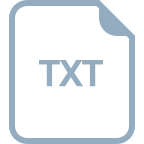
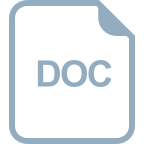



