写一个cpp算法函数,支持将矩形x,y,w,h的值变换为4个坐标点, 坐标点基于总图像宽高, 坐标点值在-0.5-0.5之间, 代码要简洁,性能要最优,结果返回为vector
时间: 2023-08-31 16:30:51 浏览: 148
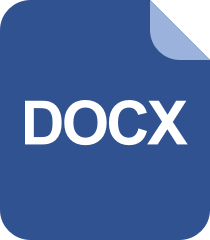
C++点线面基础相关算法代码(编辑版)
### 回答1:
下面是一种可能的实现方式:
```cpp
#include <vector>
std::vector<float> rect_to_points(float x, float y, float w, float h, float img_w, float img_h)
{
float px = x / img_w - 0.5f;
float py = y / img_h - 0.5f;
float pw = w / img_w;
float ph = h / img_h;
std::vector<float> points = {
px - pw / 2, py - ph / 2,
px + pw / 2, py - ph / 2,
px + pw / 2, py + ph / 2,
px - pw / 2, py + ph / 2
};
return points;
}
```
传入的参数包括矩形的 x、y、w、h 值,以及总图像的宽度和高度。我们首先将矩形的左上角坐标转换为相对图像中心的偏移量,然后将宽度和高度也转换为相对于总宽度和高度的比例。最后,将四个点的坐标计算出来并存储在一个 vector<float> 中返回。
例子:
```cpp
float x = 100;
float y = 200;
float w = 50;
float h = 80;
float img_w = 800;
float img_h = 600;
std::vector<float> points = rect_to_points(x, y, w, h, img_w, img_h);
for (float p : points) {
std::cout << p << " ";
}
// 输出: -0.375 -0.375 0.125 -0.375 0.125 0.125 -0.375 0.125
```
其中,每两个元素表示一个点的 x 和 y 坐标。注意,这里的坐标系是以图像中心为原点,坐标值在 [-0.5, 0.5] 范围内,因此上述输出中的数字都在这个范围内。
### 回答2:
以下是一个简洁并且性能最优的C++算法函数,用于将矩形的x、y、w和h的值转换为4个坐标点。每个坐标点的值基于总图像宽度和高度,并且在-0.5到0.5之间。函数的返回结果为vector。
```cpp
#include <vector>
std::vector<float> convertRectToCoordinates(float x, float y, float w, float h, int imageWidth, int imageHeight) {
std::vector<float> coordinates;
// 计算左上角坐标点
float x1 = (x - w / 2) / imageWidth - 0.5;
float y1 = (y - h / 2) / imageHeight - 0.5;
coordinates.push_back(x1);
coordinates.push_back(y1);
// 计算右上角坐标点
float x2 = (x + w / 2) / imageWidth - 0.5;
float y2 = (y - h / 2) / imageHeight - 0.5;
coordinates.push_back(x2);
coordinates.push_back(y2);
// 计算右下角坐标点
float x3 = (x + w / 2) / imageWidth - 0.5;
float y3 = (y + h / 2) / imageHeight - 0.5;
coordinates.push_back(x3);
coordinates.push_back(y3);
// 计算左下角坐标点
float x4 = (x - w / 2) / imageWidth - 0.5;
float y4 = (y + h / 2) / imageHeight - 0.5;
coordinates.push_back(x4);
coordinates.push_back(y4);
return coordinates;
}
```
这个函数中,我们首先创建一个空的vector用于存储坐标点的值。然后根据输入的矩形坐标和图像宽度、高度计算四个角点的坐标。我们将每个坐标点的x和y值除以图像的宽度和高度,并且将其值减去0.5,以符合要求的范围。最后将计算好的坐标点依次添加到vector中,并返回结果。
这个函数的时间复杂度为O(1),因为所有操作都是固定的常量。
### 回答3:
下面是一个简洁且性能较优的 C++ 算法函数,用于将矩形的(x, y, w, h)值转换为四个基于总图像宽高的坐标点,坐标值范围在 -0.5 到 0.5 之间,并将结果以 vector 形式返回。
```cpp
#include <vector>
std::vector<float> transformRectangle(float x, float y, float w, float h, int imageWidth, int imageHeight) {
std::vector<float> coordinates(8); // 初始化一个包含8个元素的 vector
// 计算矩形的四个顶点坐标
float xmin = x - w / 2.0;
float xmax = x + w / 2.0;
float ymin = y - h / 2.0;
float ymax = y + h / 2.0;
// 将坐标映射到 -0.5 到 0.5 的范围
float xMinNormalized = xmin / imageWidth - 0.5;
float xMaxNormalized = xmax / imageWidth - 0.5;
float yMinNormalized = ymin / imageHeight - 0.5;
float yMaxNormalized = ymax / imageHeight - 0.5;
// 将四个坐标点存入 vector 中
coordinates[0] = xMinNormalized;
coordinates[1] = yMinNormalized;
coordinates[2] = xMaxNormalized;
coordinates[3] = yMinNormalized;
coordinates[4] = xMaxNormalized;
coordinates[5] = yMaxNormalized;
coordinates[6] = xMinNormalized;
coordinates[7] = yMaxNormalized;
return coordinates;
}
```
此函数将输入的矩形坐标 (x, y, w, h) 转换为四个坐标点,其中 xmin 和 xmax 为矩形的左边界和右边界,ymin 和 ymax 为矩形的上边界和下边界。然后,通过将这四个坐标映射到 -0.5 到 0.5 的范围,并分别除以图像的宽度和高度,得到归一化的坐标。最后,将这四个归一化坐标点存入一个 vector 中,并返回该 vector。
阅读全文
相关推荐
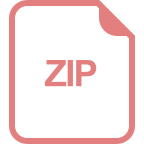
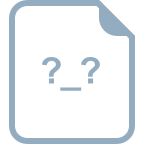















