俄罗斯方块游戏的代码
时间: 2024-02-25 10:50:08 浏览: 17
俄罗斯方块游戏是一款经典的益智游戏,玩家需要操作不同形状的方块,使其在一个矩形的游戏区域内堆叠起来,当一行被填满时,该行会被消除并得分。下面是一个简单的俄罗斯方块游戏的代码示例:
```python
import pygame
import random
# 游戏区域大小
WIDTH = 10
HEIGHT = 20
# 方块形状
SHAPES = [
[[1, 1, 1, 1]],
[[1, 1], [1, 1]],
[[1, 1, 0], [0, 1, 1]],
[[0, 1, 1], [1, 1, 0]],
[[1, 1, 1], [0, 1, 0]],
[[1, 1, 1], [1, 0, 0]],
[[1, 1, 1], [0, 0, 1]]
]
# 方块颜色
COLORS = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(255, 0, 255),
(0, 255, 255)
]
class TetrisGame:
def __init__(self):
pygame.init()
self.screen = pygame.display.set_mode((300, 600))
pygame.display.set_caption("俄罗斯方块")
self.clock = pygame.time.Clock()
self.grid = [[0] * WIDTH for _ in range(HEIGHT)]
self.current_shape = self.get_random_shape()
self.current_x = WIDTH // 2 - len(self.current_shape[0]) // 2
self.current_y = 0
self.score = 0
def get_random_shape(self):
return random.choice(SHAPES)
def draw_grid(self):
for y in range(HEIGHT):
for x in range(WIDTH):
pygame.draw.rect(self.screen, COLORS[self.grid[y][x]], (x * 30, y * 30, 30, 30), 0)
def draw_shape(self):
for y in range(len(self.current_shape)):
for x in range(len(self.current_shape[0])):
if self.current_shape[y][x]:
pygame.draw.rect(self.screen, COLORS[self.current_shape[y][x]], ((self.current_x + x) * 30, (self.current_y + y) * 30, 30, 30), 0)
def check_collision(self):
for y in range(len(self.current_shape)):
for x in range(len(self.current_shape[0])):
if self.current_shape[y][x] and (self.current_y + y >= HEIGHT or self.current_x + x < 0 or self.current_x + x >= WIDTH or self.grid[self.current_y + y][self.current_x + x]):
return True
return False
def merge_shape(self):
for y in range(len(self.current_shape)):
for x in range(len(self.current_shape[0])):
if self.current_shape[y][x]:
self.grid[self.current_y + y][self.current_x + x] = len(COLORS) - 1
def remove_full_lines(self):
full_lines = []
for y in range(HEIGHT):
if all(self.grid[y]):
full_lines.append(y)
for y in full_lines:
del self.grid[y]
self.grid.insert(0, [0] * WIDTH)
self.score += len(full_lines)
def game_over(self):
pygame.quit()
def run(self):
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
self.game_over()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.current_x -= 1
if self.check_collision():
self.current_x += 1
elif event.key == pygame.K_RIGHT:
self.current_x += 1
if self.check_collision():
self.current_x -= 1
elif event.key == pygame.K_DOWN:
self.current_y += 1
if self.check_collision():
self.current_y -= 1
elif event.key == pygame.K_UP:
rotated_shape = list(zip(*self.current_shape[::-1]))
if not self.check_collision(rotated_shape):
self.current_shape = rotated_shape
self.current_y += 1
if self.check_collision():
self.current_y -= 1
self.merge_shape()
self.remove_full_lines()
self.current_shape = self.get_random_shape()
self.current_x = WIDTH // 2 - len(self.current_shape[0]) // 2
self.current_y = 0
if self.check_collision():
self.game_over()
self.screen.fill((255, 255, 255))
self.draw_grid()
self.draw_shape()
pygame.display.update()
self.clock.tick(10)
if __name__ == "__main__":
game = TetrisGame()
game.run()
```
这是一个使用Python和Pygame库实现的简单俄罗斯方块游戏代码。代码中使用了一个二维数组来表示游戏区域,通过不断更新方块的位置和状态来实现游戏的进行。你可以根据自己的需求进行修改和扩展。
相关推荐
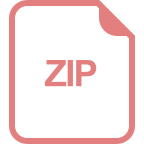














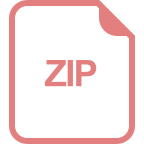