#include<stdio.h> #include<malloc.h> typedef struct _student_info_ { char s_id[16]; //学号 char s_name[32]; //姓名 int s_score[3]; //三科成绩 }student_info; void input(student_info *L) { scanf("%s %s %d %d %d", L->s_id, L->s_name, &(L->s_score[0]), &(L->s_score[1]), &(L->s_score[2])); } void print(student_info *L) { printf("%s,%s,%d,%d,%d\n", L->s_id, L->s_name, L->s_score[0], L->s_score[1], L->s_score[2]); } int main() { student_info *list; int n = 0, i = 0; scanf("%d", &n); while(getchar() != '\n') continue; if(n > 0 && n < 100) list = (student_info *)malloc(sizeof(student_info)*n); else return 0; for(i = 0; i < n; i++) { input(&list[i]); //一行一行获取,忽略一行多余的数据 while(getchar() != '\n') continue; } for(i = 0; i < n; i++) { print(&list[i]); } free(list); return 0; }给以上代码添加注释
时间: 2024-04-16 13:24:03 浏览: 75
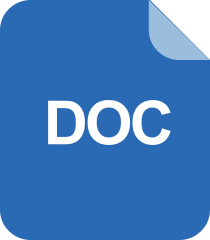
栈和队列的基础上设计模拟停车场管理程序 停车场管理问题 #include<stdio.h> #include<stdlib.h> struct { char status; int num; int time; }a; /*命令的结构*/ typedef struct{ int num; int time; }Element; struct { Element *base; Element *top; in
```c
#include <stdio.h>
#include <malloc.h>
typedef struct _student_info_
{
char s_id[16]; // 学号
char s_name[32]; // 姓名
int s_score[3]; // 三科成绩
} student_info;
void input(student_info *L)
{
scanf("%s %s %d %d %d", L->s_id, L->s_name, &(L->s_score[0]), &(L->s_score[1]), &(L->s_score[2]));
}
void print(student_info *L)
{
printf("%s,%s,%d,%d,%d\n", L->s_id, L->s_name, L->s_score[0], L->s_score[1], L->s_score[2]);
}
int main()
{
student_info *list;
int n = 0, i = 0;
scanf("%d", &n);
while (getchar() != '\n')
continue;
if (n > 0 && n < 100)
list = (student_info *)malloc(sizeof(student_info) * n); // 动态分配内存空间
else
return 0;
for (i = 0; i < n; i++)
{
input(&list[i]);
// 一行一行获取,忽略一行多余的数据
while (getchar() != '\n')
continue;
}
for (i = 0; i < n; i++)
{
print(&list[i]);
}
free(list); // 释放内存空间
return 0;
}
```
这是一个简单的学生成绩管理程序。程序通过动态分配内存,存储学生的学号、姓名和三科成绩,并可以输入和输出学生信息。请注意,此代码没有进行错误处理和输入验证。
阅读全文
相关推荐
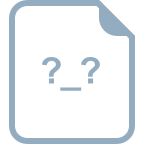
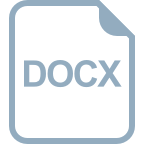
















