a 已知x1,y1,theta1 b 已知x2,y2判断b在a的左右给出python程序
时间: 2024-03-17 09:44:50 浏览: 66
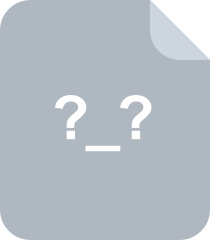
python双屏判断程序
假设 `a` 和 `b` 的格式分别为 `(x1, y1, theta1)` 和 `(x2, y2)`,其中 `theta1` 表示极角(也就是点 `a` 与原点的连线与 x 轴正方向的夹角),则可以按照以下方式判断点 `b` 在点 `a` 的左侧还是右侧:
```python
import math
# 定义点 a 和点 b
a = (1, 2, math.pi/4) # 极角为 pi/4
b = (3, 4)
# 计算点 b 相对于点 a 的极角
theta2 = math.atan2(b[1]-a[1], b[0]-a[0])
# 将极角转换为角度,并归一化到 [0, 360) 的范围
angle_a = math.degrees(a[2]) % 360
angle_b = math.degrees(theta2) % 360
# 判断点 b 在点 a 的左侧还是右侧
if (angle_b > angle_a and angle_b < (angle_a + 180)) or (angle_b < (angle_a - 180)):
print("点 b 在点 a 的左侧")
else:
print("点 b 在点 a 的右侧")
```
这里使用了 Python 的 `math` 模块中的 `atan2` 函数来计算点 `b` 相对于点 `a` 的极角。然后将极角转换为角度,并归一化到 `[0, 360)` 的范围内,最后根据角度的大小关系判断点 `b` 在点 `a` 的左侧还是右侧。
阅读全文
相关推荐
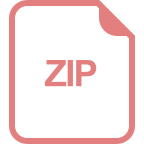
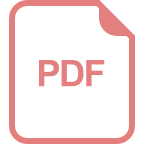

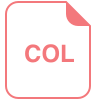













