基于openmv,用MicroPython写一段用WIFI无线图传的程序
时间: 2023-09-19 09:06:46 浏览: 159
### 回答1:
很高兴回答你的问题。以下是基于OpenMV和MicroPython编写的使用WiFi无线图传的示例程序:
```python
import network
import usocket as socket
import ustruct as struct
import time
import sensor
import image
import json
# 设置WiFi连接参数
SSID='your_wifi_ssid'
KEY='your_wifi_password'
# 初始化网络连接
print("连接WiFi...")
sta_if = network.WLAN(network.STA_IF)
sta_if.active(True)
sta_if.connect(SSID, KEY)
while not sta_if.isconnected():
time.sleep_ms(500)
print('WiFi连接成功')
print('网络配置信息:', sta_if.ifconfig())
# 初始化图像传输服务器
sock = socket.socket()
sock.bind(('', 8000))
sock.listen(1)
print('图像传输服务器已经开启')
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time=2000)
# 开始循环图像传输
while True:
try:
# 接收客户端的连接请求
client, addr = sock.accept()
print('已连接:', addr)
# 采集图像
img = sensor.snapshot()
# 图像编码为JPG格式
img = img.compress(quality=30)
# 发送图像大小
size = struct.pack('<L', img.size())
client.send(size)
# 发送图像数据
client.sendall(img)
# 关闭连接
client.close()
except KeyboardInterrupt:
# 中断程序
print('程序已中断')
break
# 断开WiFi连接
sta_if.disconnect()
print('WiFi连接已经断开')
```
请注意,此程序仅供参考,具体的实现方式可能会因硬件、网络环境等因素而略有不同。希望对您有所帮助。
### 回答2:
基于OpenMV,结合MicroPython编写一段使用WIFI无线图传的程序如下:
```python
import network
from machine import UART
import sensor
import image
import time
import ujson
# 创建WIFI连接
def connect_wifi(ssid, password):
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
if not wlan.isconnected():
wlan.connect(ssid, password)
while not wlan.isconnected():
pass
print('已连接WIFI')
print('网络配置:', wlan.ifconfig())
# 初始化串口
uart = UART(3, 9600) # 通过串口与OpenMV相连
# 连接WIFI
wifi_ssid = "your_wifi_ssid"
wifi_password = "your_wifi_password"
connect_wifi(wifi_ssid, wifi_password)
while True:
if uart.any():
json_data = uart.readline()
# 解析接收到的JSON数据
try:
data = ujson.loads(json_data)
image_data = data["image"]
# 解码图像数据
img = image.ImageIO(image_data)
img = img.to_image()
img.save("received_image.jpg") # 将接收到的图像保存为文件
print("已接收到图像并保存")
except Exception as e:
print("无法解析JSON数据:", str(e))
else:
print("等待接收图像数据...")
time.sleep(1)
```
以上程序是一个简单的使用WIFI无线图传的示例。它通过串口与OpenMV相连,并通过WIFI接收图像数据。在程序中,我们首先创建了一个连接WIFI的函数`connect_wifi()`。然后我们初始化串口,和OpenMV建立连接,并设置波特率为9600。接着使用`connect_wifi()`函数连接到指定的WIFI网络。
在主循环中,我们使用`uart.any()`函数检查串口是否有数据接收。如果有数据接收,我们将接收到的JSON数据进行解析,并获取图像数据(在JSON中以字符串形式存储)。然后,我们将图像数据解码为图像对象,并保存为文件。如果无法解析JSON数据,将会打印出错误信息。如果串口没有数据接收,将会输出等待接收图像数据的提示信息。
以上程序仅作为示例,实际应用中还需要根据具体情况进行修改和优化。例如,可能需要加入数据校验和重传机制,以确保数据传输的可靠性。
相关推荐
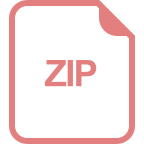
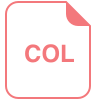
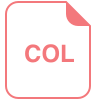
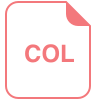
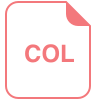
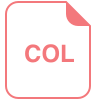









