单链表中存放着n个字符,设计算法,判断该字符串中是否有中心对称关系。例如: xyzzyx、xyzyx都算是中心对称的字符串,用c语言实现运算
时间: 2024-09-13 20:06:51 浏览: 57
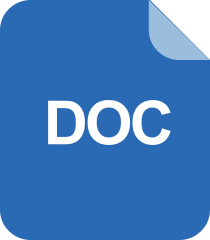
数据结构实验
中心对称字符串是指一个字符串从前向后读和从后向前读是相同的。例如:"xyzzyx" 和 "xyzyx" 都满足中心对称的条件。可以通过比较字符串的前半部分和翻转后的后半部分来判断是否中心对称。下面是一个简单的C语言算法实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 函数声明
int isPalindrome(char *str);
int main() {
char str[] = "xyzzyx"; // 示例字符串
if (isPalindrome(str)) {
printf("字符串 \"%s\" 是中心对称的。\n", str);
} else {
printf("字符串 \"%s\" 不是中心对称的。\n", str);
}
return 0;
}
// 函数定义:判断字符串是否为中心对称
int isPalindrome(char *str) {
int len = strlen(str);
int start = 0;
int end = len - 1;
while (start <= end) {
if (str[start] != str[end]) {
return 0; // 发现不匹配,不是中心对称字符串
}
start++;
end--;
}
return 1; // 所有字符都匹配,是中心对称字符串
}
```
在这个算法中,`isPalindrome` 函数接收一个字符串参数,并使用双指针技术从字符串的两端开始比较字符。如果遇到不匹配的字符,则立即返回0表示字符串不是中心对称的。如果所有字符都匹配,则返回1表示字符串是中心对称的。
阅读全文
相关推荐
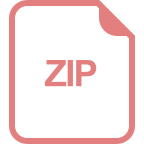
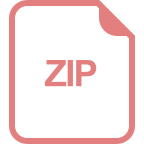











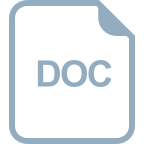


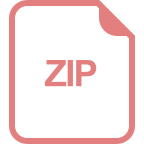