编写C++程序实现顺序栈的初始化、判断栈空否、入栈和出栈等
时间: 2023-05-30 10:03:06 浏览: 53
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int top; // 栈顶指针,初始值为-1
} SeqStack;
// 初始化栈
void initStack(SeqStack *s) {
s->top = -1;
}
// 判断栈空
int isEmpty(SeqStack *s) {
return s->top == -1;
}
// 判断栈满
int isFull(SeqStack *s) {
return s->top == MAX_SIZE - 1;
}
// 入栈
int push(SeqStack *s, int x) {
if (isFull(s)) {
printf("Stack overflow!\n");
return 0;
}
s->top++;
s->data[s->top] = x;
return 1;
}
// 出栈
int pop(SeqStack *s, int *x) {
if (isEmpty(s)) {
printf("Stack underflow!\n");
return 0;
}
*x = s->data[s->top];
s->top--;
return 1;
}
// 测试
int main() {
SeqStack s;
initStack(&s);
printf("isEmpty: %d\n", isEmpty(&s));
push(&s, 1);
push(&s, 2);
push(&s, 3);
int x;
pop(&s, &x);
printf("pop: %d\n", x);
printf("isEmpty: %d\n", isEmpty(&s));
return 0;
}
相关推荐
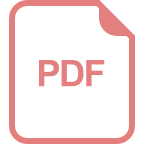
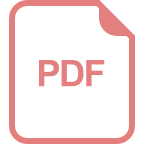












