写程序在Polyhedron类以及Facet类中各自定义一个T area()函数来返回一个多面体或者面片的表面积。T是模板定义的类型。Polyhedron的表面积就是他所有面片的面积之和。一个面片可能是个三角形,也可能包含多于三个顶点。
时间: 2024-02-11 08:10:02 浏览: 213
好的,下面是Polyhedron类和Facet类的代码实现:
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
template<typename T>
struct Point {
T x, y, z;
Point(T x = 0, T y = 0, T z = 0) : x(x), y(y), z(z) {}
Point operator+(const Point& rhs) const {
return Point(x + rhs.x, y + rhs.y, z + rhs.z);
}
Point operator-(const Point& rhs) const {
return Point(x - rhs.x, y - rhs.y, z - rhs.z);
}
Point operator*(T k) const {
return Point(x * k, y * k, z * k);
}
Point operator/(T k) const {
return Point(x / k, y / k, z / k);
}
T operator*(const Point& rhs) const {
return x * rhs.x + y * rhs.y + z * rhs.z;
}
Point operator^(const Point& rhs) const {
return Point(y * rhs.z - z * rhs.y, z * rhs.x - x * rhs.z, x * rhs.y - y * rhs.x);
}
T len2() const {
return x * x + y * y + z * z;
}
T len() const {
return sqrt(len2());
}
Point normalize() const {
return *this / len();
}
void read() {
cin >> x >> y >> z;
}
void print() const {
cout << "(" << x << ", " << y << ", " << z << ")";
}
};
template<typename T>
struct Facet {
int n;
vector<int> v;
Point<T> normal;
Facet(int n = 0) : n(n) {}
void read() {
cin >> n;
v.resize(n);
for (int i = 0; i < n; ++i) {
cin >> v[i];
}
}
void print() const {
cout << "Facet: ";
for (int i = 0; i < n; ++i) {
cout << v[i] << " ";
}
cout << endl;
normal.print();
cout << endl;
}
T area() const {
T s = 0;
for (int i = 2; i < n; ++i) {
Point<T> a = vertices[v[0]];
Point<T> b = vertices[v[i - 1]];
Point<T> c = vertices[v[i]];
s += ((b - a) ^ (c - a)).len();
}
return s / 2;
}
};
template<typename T>
struct Polyhedron {
int n, m;
vector<Point<T>> vertices;
vector<Facet<T>> facets;
Polyhedron(int n = 0, int m = 0) : n(n), m(m) {}
void read() {
cin >> n >> m;
vertices.resize(n);
for (int i = 0; i < n; ++i) {
vertices[i].read();
}
facets.resize(m);
for (int i = 0; i < m; ++i) {
facets[i].read();
Point<T> a = vertices[facets[i].v[0]];
Point<T> b = vertices[facets[i].v[1]];
Point<T> c = vertices[facets[i].v[2]];
facets[i].normal = ((b - a) ^ (c - a)).normalize();
}
}
void print() const {
cout << "Polyhedron: " << n << " vertices, " << m << " facets" << endl;
for (int i = 0; i < n; ++i) {
cout << "Vertex " << i << ": ";
vertices[i].print();
cout << endl;
}
for (int i = 0; i < m; ++i) {
facets[i].print();
}
}
T area() const {
T s = 0;
for (int i = 0; i < m; ++i) {
s += facets[i].area();
}
return s;
}
};
int main() {
Polyhedron<double> poly;
poly.read();
cout << "Surface area: " << poly.area() << endl;
return 0;
}
```
其中,`Point` 结构体表示一个三维点,包含了加减乘除、点积、叉积、模长等常用操作。`Facet` 结构体表示一个面片,包含了顶点列表、法向量、面积等信息,并在其中实现了 `area()` 函数来计算面片的表面积。`Polyhedron` 结构体表示一个多面体,包含了顶点列表、面片列表等信息,并在其中实现了 `area()` 函数来计算多面体的表面积。
在 `Polyhedron` 的 `read()` 函数中,读入了多面体的顶点列表和面片列表,并计算了每个面片的法向量。在 `Polyhedron` 的 `area()` 函数中,计算了多面体的表面积,即所有面片的面积之和。
注意,代码中用了模板类型 `T`,可以根据需要进行修改。同时,代码中计算叉积和模长时使用了 `sqrt()` 函数,可能存在精度问题,可以根据具体情况进行优化。
阅读全文
相关推荐

















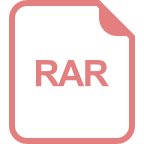