python读取tsp文件
时间: 2023-07-28 07:09:05 浏览: 99
要读取 TSP 文件,你可以使用 Python 中的文件操作函数。以下是一个简单的示例代码,它假设 TSP 文件中每行包含一个城市的坐标(x,y):
```python
def read_tsp_file(filename):
cities = []
with open(filename, 'r') as file:
for line in file:
x, y = map(float, line.split())
cities.append((x, y))
return cities
# 示例用法
filename = 'cities.tsp'
cities = read_tsp_file(filename)
print(cities)
```
在上面的示例中,`read_tsp_file` 函数接受一个文件名作为参数,并逐行读取文件内容。它将每行分割为两个浮点数,并将它们作为元组添加到 `cities` 列表中。最后,函数返回城市列表。
你需要将 `filename` 替换为你实际的 TSP 文件路径。确保文件的格式与代码中的假设一致,以避免错误。
相关问题
python怎么读取tsp文件
可以使用Python的pandas库来读取.tsp文件。在读取文件时,可以使用read_csv()函数,并设置适当的参数来处理文件的格式。下面是一个使用pandas读取.tsp文件的示例代码:
import pandas as pd
df = pd.read_csv("文件路径", sep=" ", skiprows=行数, header=None, error_bad_lines=False)
其中,"文件路径"是你要读取的.tsp文件的路径。sep参数指定了文件中各列之间的分隔符,这里是空格。skiprows参数指定了要跳过的行数,通常是文件中的元数据行。header参数指定了是否将第一行作为列名,这里设置为None表示没有列名。error_bad_lines参数设置为False时,会忽略错误行并跳过读取。
遗传算法解决tsp问题文件操作
遗传算法可以用于解决TSP问题,其中文件操作是指读取和处理TSP问题的输入文件。具体步骤如下:
1. 读取输入文件:从文件中读取TSP问题的相关信息,包括城市数量、城市之间的距离矩阵等。
2. 初始化种群:根据城市数量,随机生成初始的种群,每个个体代表一条路径。
3. 评估适应度:计算每个个体的适应度,即路径的总长度。适应度越好,表示路径越短。
4. 选择操作:根据适应度值,选择一部分个体作为父代,用于产生下一代个体。
5. 交叉操作:对选中的父代个体进行交叉操作,生成新的子代个体。
6. 变异操作:对子代个体进行变异操作,引入随机性,增加种群的多样性。
7. 更新种群:将父代和子代个体合并,形成新的种群。
8. 重复步骤3-7,直到达到停止条件(例如达到最大迭代次数或找到最优解)。
9. 输出结果:输出最优解的路径和路径长度。
下面是一个示例代码,演示了如何使用遗传算法解决TSP问题的文件操作:
```python
import numpy as np
# 读取输入文件
def read_input_file(file_path):
# 读取文件内容并解析
# 返回城市数量和距离矩阵
pass
# 初始化种群
def initialize_population(population_size, city_count):
# 随机生成初始种群
# 每个个体代表一条路径
pass
# 计算适应度
def calculate_fitness(population, distance_matrix):
# 计算每个个体的适应度(路径长度)
pass
# 选择操作
def selection(population, fitness):
# 根据适应度值选择父代个体
pass
# 交叉操作
def crossover(parent1, parent2):
# 对选中的父代个体进行交叉操作,生成子代个体
pass
# 变异操作
def mutation(individual):
# 对子代个体进行变异操作,引入随机性
pass
# 更新种群
def update_population(parents, offspring):
# 将父代和子代个体合并,形成新的种群
pass
# 主函数
def tsp_ga(file_path, population_size, max_iterations):
# 读取输入文件
city_count, distance_matrix = read_input_file(file_path)
# 初始化种群
population = initialize_population(population_size, city_count)
# 迭代求解
for i in range(max_iterations):
# 计算适应度
fitness = calculate_fitness(population, distance_matrix)
# 选择操作
parents = selection(population, fitness)
# 交叉操作
offspring = crossover(parents)
# 变异操作
offspring = mutation(offspring)
# 更新种群
population = update_population(parents, offspring)
# 输出结果
best_individual = population[np.argmin(fitness)]
best_path = decode_path(best_individual)
best_length = fitness[np.argmin(fitness)]
print("Best path: ", best_path)
print("Best length: ", best_length)
# 调用主函数
tsp_ga("input.txt", 100, 1000)
```
相关推荐
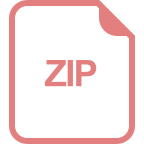
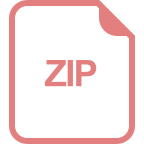












