用c++写一段代码实现 多项式的相加 1、用顺序表来存放多项式的系数和指数 2、完成两个多项式的相加,将合并后的系数和指数存放在新的顺序表中
时间: 2024-09-29 16:11:15 浏览: 51
在C++中,我们可以使用`std::pair`结构体来存储多项式的系数和指数,然后创建一个自定义的数据结构,如`Polynomial`,用于顺序列表表示。下面是一个简单的实现:
```cpp
#include <iostream>
#include <vector>
#include <utility>
// 定义多项式元素
using CoeffAndExp = std::pair<int, int>;
// 多项式数据结构
class Polynomial {
private:
std::vector<CoeffAndExp> coefficients;
public:
// 添加一个项到多项式
void addTerm(int coeff, int exp) {
coefficients.push_back(std::make_pair(coeff, exp));
}
// 合并两个多项式
void add(Polynomial other) {
for (const auto& term : other.coefficients) {
if (coefficients.end() == find(coefficients.begin(), coefficients.end(), term)) {
coefficients.push_back(term);
}
}
}
// 打印多项式
void print() {
std::cout << "Polynomial: ";
for (const auto& term : coefficients) {
std::cout << term.first << "x^" << term.second << " + ";
}
// 移除最后一个 '+ '
if (!coefficients.empty()) {
coefficients.back().first--;
}
std::cout << "\n";
}
};
int main() {
Polynomial poly1;
poly1.addTerm(5, 0); // 5x^0
poly1.addTerm(3, 2); // 3x^2
Polynomial poly2;
poly2.addTerm(2, 1); // 2x^1
poly2.addTerm(-1, 3); // -1x^3
// 将poly2添加到poly1上
poly1.add(poly2);
// 打印结果
poly1.print();
return 0;
}
```
在这个示例中,我们首先定义了`CoeffAndExp`来存储系数和指数,然后在`Polynomial`类中实现了添加项(`addTerm`)和合并两个多项式(`add`)的方法。`main()`函数展示了如何创建和操作这两个多项式。
阅读全文
相关推荐












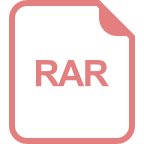




