python退火算法
时间: 2023-08-21 10:14:57 浏览: 47
Python中的退火算法(Simulated Annealing)是一种全局优化算法,用于在搜索空间中寻找最优解。它模拟了固体退火过程中的原子结构热运动,通过逐渐降低温度来减少系统能量,最终达到稳定状态。
在Python中,你可以使用以下步骤实现退火算法:
1. 定义问题的目标函数,即需要最小化或最大化的指标。
2. 初始化搜索空间中的一个随机解作为当前解。
3. 设置初始温度和退火率。
4. 迭代过程中,通过随机调整当前解来生成新解,并计算目标函数值之间的差异。
5. 根据Metropolis准则,决定是否接受新解作为当前解。如果新解更优,则接受;否则,根据一定概率接受较差的解。
6. 降低温度,使得接受较差解的概率逐渐减小。
7. 重复步骤4-6,直到满足停止条件(例如达到最大迭代次数或温度降低到一定程度)。
需要注意的是,退火算法的效果高度依赖于参数的选择和问题本身的特性。因此,根据具体问题的不同,可能需要进行参数调整或引入问题特定的优化策略。
在Python中,你可以使用一些第三方库,如SciPy或PySwarms,来实现退火算法。这些库提供了现成的优化函数和工具,简化了算法的实现过程。你可以根据自己的需求选择适合的库,并参考其文档以了解更多详细信息。
相关问题
python 退火算法
Python中的退火算法是一种全局优化算法,用于在大规模搜索空间中找到一个接近全局最优解的解。它模拟了金属退火过程中的原理,通过随机搜索和概率接受较差解的方式,在搜索空间中逐渐找到更优的解。
以下是一个简单的示例代码来实现退火算法:
```python
import random
import math
def cost_function(solution):
# 计算适应度函数(成本函数)值,根据具体问题而定
pass
def generate_neighbor(solution):
# 生成邻居解,根据具体问题而定
pass
def acceptance_probability(cost, new_cost, temperature):
if new_cost < cost:
return 1
return math.exp((cost - new_cost) / temperature)
def simulated_annealing(initial_solution, initial_temperature, final_temperature, cooling_rate):
current_solution = initial_solution
best_solution = initial_solution
current_temperature = initial_temperature
while current_temperature > final_temperature:
new_solution = generate_neighbor(current_solution)
current_cost = cost_function(current_solution)
new_cost = cost_function(new_solution)
if acceptance_probability(current_cost, new_cost, current_temperature) > random.random():
current_solution = new_solution
if cost_function(current_solution) < cost_function(best_solution):
best_solution = current_solution
current_temperature *= cooling_rate
return best_solution
# 使用示例
initial_solution = ... # 初始解,据具体问题而定
initial_temperature = ... # 初始温度
final_temperature = ... # 最终温度
cooling_rate = ... # 降温速率
best_solution = simulated_annealing(initial_solution, initial_temperature, final_temperature, cooling_rate)
print(best_solution)
```
其中,`cost_function`函数用于计算解的成本(适应度)函数值,`generate_neighbor`函数用于生成邻居解,`acceptance_probability`函数用于计算接受新解的概率。
在退火算法中,初始温度和降温速率的选择对算法的性能有一定的影响。通常情况下,初始温度应该足够高,以便在搜索空间中进行充分的探索,而降温速率应该足够慢,以充分利用退火的过程。
请注意,以上代码只是一个简单示例,实际问题中需要根据具体情况进行相应的修改和调整。
python 退火算法碉堡
你可以使用Python的Simulated Annealing库来实现退火算法。该库可以帮助你快速实现退火算法的代码,而无需自己从头开始编写。
你可以在Python中使用`pip`命令安装Simulated Annealing库。打开终端或命令提示符,运行以下命令:
```
pip install simulated-annealing
```
安装完成后,你可以在Python脚本中导入并使用该库。以下是一个简单示例:
```python
from simulated_annealing import Annealer
# 定义问题的初始状态
initial_state = [0, 0, 0, 0]
# 定义问题的目标函数
def objective_function(state):
# 这里是你要优化的目标函数,根据实际情况进行替换
return state[0] ** 2 + state[1] ** 2 + state[2] ** 2 + state[3] ** 2
# 创建一个退火模拟器
annealer = Annealer(objective_function)
# 运行退火算法
best_state, best_value = annealer.anneal(initial_state)
# 输出结果
print("Best State:", best_state)
print("Best Value:", best_value)
```
在上述示例中,我们定义了一个包含4个变量的问题,并给出了一个初始状态。然后,我们定义了一个目标函数,根据实际情况进行替换。接下来,我们创建了一个退火模拟器,并运行退火算法。最后,我们输出了找到的最佳状态和对应的目标函数值。
希望这个示例能帮助你开始使用退火算法。记得根据你的具体问题进行适当调整和修改。
相关推荐
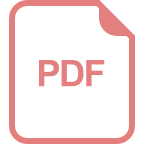











