1、 用递归算法找到该二叉树中节点data域的最大值,并输出 2、 用递归算法计算该二叉树中所有节点的data域之和 3、 用递归算法统计出该二叉树的高度 4、 用递归算法该二叉树中单分支节点的数量
时间: 2024-12-08 15:24:33 浏览: 7
1. 要用递归算法找二叉树中节点data域的最大值,可以从根节点开始,比较当前节点的数据和左右子节点(如果存在)的最大值,返回较大者。递归终止条件是在叶子节点,此时最大值即为该节点的数据。以下是伪代码:
```python
def findMax(node):
if node is None:
return float('-inf')
else:
max_val = max(node.data, findMax(node.left), findMax(node.right))
return max_val
```
2. 计算所有节点的data域之和同样从根节点开始,累加并递归地处理子节点。递归终止条件也是在叶子节点。伪代码如下:
```python
def sumNodes(node):
if node is None:
return 0
else:
return node.data + sumNodes(node.left) + sumNodes(node.right)
```
3. 算法求二叉树高度(深度)也从根节点开始,高度为1加上左子树和右子树的最高高度中的较大值。递归终止条件是在叶子节点,高度为0。伪代码:
```python
def height(node):
if node is None:
return 0
else:
left_height = height(node.left)
right_height = height(node.right)
return max(left_height, right_height) + 1
```
4. 统计单分支节点(只有一个子节点的节点)的数量,我们需要在访问每个节点时检查它是否有两个子节点。伪代码:
```python
def countSingleBranchNodes(node, single_branch_count=0):
if node is None:
return single_branch_count
elif node.left and not node.right:
single_branch_count += 1
elif not node.left and node.right:
single_branch_count += 1
return countSingleBranchNodes(node.left, single_branch_count) + \
countSingleBranchNodes(node.right, single_branch_count)
```
阅读全文
相关推荐
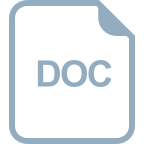
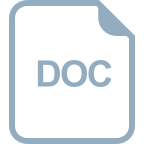
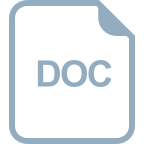
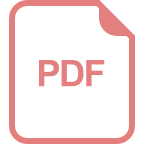












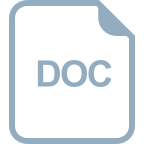