KeyError Traceback (most recent call last) Cell In[6], line 10 8 history1 = model1.fit(x_train, y_train, epochs=epochs, batch_size=batch_size, validation_data=(x_test,y_test)) 9 # 再训练model2 ---> 10 history2 = model2.fit(x_train, y_train, epochs=epochs, batch_size=batch_size, validation_data=(x_test,y_test)) File ~\anaconda3\lib\site-packages\keras\src\utils\traceback_utils.py:70, in filter_traceback.<locals>.error_handler(*args, **kwargs) 67 filtered_tb = _process_traceback_frames(e.traceback) 68 # To get the full stack trace, call: 69 # tf.debugging.disable_traceback_filtering() ---> 70 raise e.with_traceback(filtered_tb) from None 71 finally: 72 del filtered_tb File ~\AppData\Local\Temp_autograph_generated_filejh6by608.py:15, in outer_factory.<locals>.inner_factory.<locals>.tf__train_function(iterator) 13 try: 14 do_return = True ---> 15 retval = ag__.converted_call(ag__.ld(step_function), (ag__.ld(self), ag__.ld(iterator)), None, fscope) 16 except: 17 do_return = False KeyError: in user code: 'The optimizer cannot recognize variable dense_1/kernel:0. This usually means you are trying to call the optimizer to update different parts of the model separately. Please call `optimizer.build(variables)` with the full list of trainable variables before the training loop or use legacy optimizer `tf.keras.optimizers.legacy.SGD.
时间: 2024-04-17 07:29:35 浏览: 45
这个错误通常是由于在训练不同的模型时,优化器无法识别变量导致的。解决此问题的一个方法是在训练循环之前,使用 `optimizer.build(variables)` 方法传递完整的可训练变量列表,或者使用旧版优化器 `tf.keras.optimizers.legacy.SGD`。这样做将帮助优化器识别并更新所有需要更新的变量。
在你的代码中,你需要确保在训练 `model2` 之前,已经调用了 `optimizer.build(variables)` 或者使用了旧版优化器。这样可以避免出现这个错误。
相关问题
Traceback (most recent call last):clf.fit(X_train, y_train)
这是一个 Python 代码中的错误信息,提示在执行 `clf.fit(X_train, y_train)` 这一行代码时出现了错误。具体的错误类型和错误信息需要查看完整的错误信息才能确定。常见的错误包括语法错误、变量未定义、数据类型不匹配、函数参数错误等。在定位问题时,可以逐行检查代码,确保语法正确并且变量赋值正确。此外,也可以使用 Python 的调试工具来帮助定位错误。
>>> model = tf.keras.models.Sequential([ ... tf.keras.layers.Dense(64, activation='relu'), ... tf.keras.layers.Dense(10, activation='softmax') ... ]) >>> tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir="./logs") >>> model.fit(x_train, y_train, epochs=10, callbacks=[tensorboard_callback]) Traceback (most recent call last): File "<stdin>", line 1, in <module> NameError: name 'x_train' is not defined
这个错误提示是因为在运行这段代码之前没有定义变量 x_train。x_train 和 y_train 应该是训练模型所需的输入数据和对应的标签。你需要先定义这两个变量并将它们传递给 model.fit() 方法。例如:
```
import tensorflow as tf
# 定义 x_train 和 y_train
x_train = ...
y_train = ...
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
tensorboard_callback = tf.keras.callbacks.TensorBoard(log_dir="./logs")
model.fit(x_train, y_train, epochs=10, callbacks=[tensorboard_callback])
```
你需要将 `...` 替换为你自己的数据和标签。
相关推荐
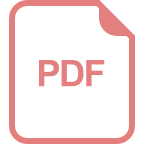
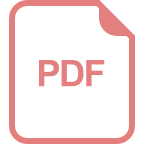
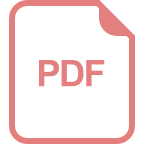













