用EasyX绘制任意个多边形,鼠标左键绘制,过程中显示路径,绘制结束后,填充多边形内部,鼠标邮件结束绘制,将已经绘制的保存到文件中
时间: 2024-04-05 16:32:49 浏览: 88
这是一个比较复杂的问题,需要使用C++和EasyX图形库进行编程。以下是一个简单的实现,代码中会有注释解释每个部分的作用:
```c++
#include <graphics.h>
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
// 存储多边形的点
vector<POINT> points;
// 保存多边形的函数
void savePolygonToFile(string filename) {
ofstream outFile(filename);
if (outFile.is_open()) {
// 保存顶点数量
outFile << points.size() << endl;
// 保存每个顶点的坐标
for (int i = 0; i < points.size(); i++) {
outFile << points[i].x << " " << points[i].y << endl;
}
outFile.close();
}
}
int main() {
initgraph(640, 480);
// 绘制多边形的过程
bool isDrawing = true;
while (isDrawing) {
// 鼠标左键按下
if (MouseHit()) {
MOUSEMSG msg = GetMouseMsg();
if (msg.uMsg == WM_LBUTTONDOWN) {
// 添加新的顶点到多边形中
POINT p = { msg.x, msg.y };
points.push_back(p);
// 显示已经绘制的路径
if (points.size() > 1) {
line(points[points.size() - 2].x, points[points.size() - 2].y, p.x, p.y);
}
}
// 鼠标右键按下
else if (msg.uMsg == WM_RBUTTONDOWN) {
// 绘制多边形的最后一条边
line(points[0].x, points[0].y, points[points.size() - 1].x, points[points.size() - 1].y);
// 填充多边形内部
setfillcolor(RGB(255, 0, 0));
fillpolygon(points.data(), points.size());
// 结束绘制
isDrawing = false;
}
}
}
// 保存多边形到文件中
savePolygonToFile("polygon.txt");
getch();
closegraph();
return 0;
}
```
这段代码使用EasyX图形库绘制多边形,并且将多边形的顶点保存到文件中。需要注意的是,这段代码中没有实现多边形的编辑功能,如果需要实现编辑功能,可以在绘制多边形的过程中使用鼠标右键删除最后一个顶点,并重新绘制多边形。
阅读全文
相关推荐





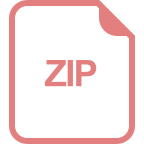
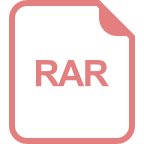
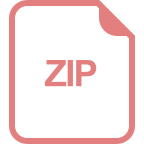
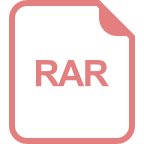
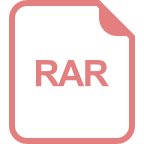
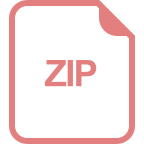
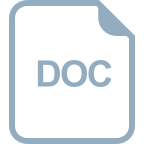



