任务:使用EasyX库设计一个绘制多边形的程序。并且满足以下要求: 1、 可以会制任意个多边形;2、过程中要显示绘制路径,绘制结束后 ,填充多边形内部; 3、能够将已绘制完成的多边形坐标保存到文件中,并且可以从文件中读取已保存的多边形坐标,重新显示在界面上。
时间: 2024-03-21 16:37:53 浏览: 12
好的,我可以帮你解决这个问题。
首先,你需要安装EasyX库,并且在代码中引入EasyX库的头文件。
以下是实现绘制多边形的程序代码:
```c++
#include <graphics.h>
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
int main()
{
vector<vector<pair<int, int>>> polygons; // 保存所有多边形的坐标
vector<pair<int, int>> points; // 保存当前多边形的坐标
bool is_drawing = false; // 是否正在绘制多边形
bool is_finished = false; // 当前多边形是否绘制完成
int init_x = 0, init_y = 0; // 鼠标按下时的坐标
int prev_x = 0, prev_y = 0; // 鼠标上一次移动时的坐标
int curr_x = 0, curr_y = 0; // 鼠标当前的坐标
initgraph(640, 480); // 初始化窗口
while (true)
{
if (kbhit() && getch() == 27) // 如果按下ESC键退出程序
break;
if (GetAsyncKeyState(VK_LBUTTON) & 0x8000) // 如果鼠标左键按下
{
curr_x = mousex();
curr_y = mousey();
if (!is_drawing) // 如果当前没有在绘制多边形
{
init_x = prev_x = curr_x;
init_y = prev_y = curr_y;
is_drawing = true;
is_finished = false;
points.clear();
points.push_back(make_pair(curr_x, curr_y));
setcolor(COLOR::BLUE);
line(init_x, init_y, curr_x, curr_y); // 绘制起始点
}
else // 如果当前正在绘制多边形
{
if (prev_x != curr_x || prev_y != curr_y) // 如果鼠标有移动
{
setcolor(COLOR::BLUE);
line(prev_x, prev_y, curr_x, curr_y); // 绘制当前线段
prev_x = curr_x;
prev_y = curr_y;
points.push_back(make_pair(curr_x, curr_y));
}
}
}
else if (is_drawing && !is_finished) // 如果鼠标左键松开且当前多边形尚未绘制完成
{
if (points.size() > 2 && curr_x == init_x && curr_y == init_y) // 如果多边形已经绘制完成
{
setcolor(COLOR::BLUE);
line(prev_x, prev_y, init_x, init_y); // 绘制最后一条线段
is_finished = true;
is_drawing = false;
polygons.push_back(points);
}
else // 如果多边形未绘制完成
{
setcolor(COLOR::BLUE);
line(prev_x, prev_y, init_x, init_y); // 绘制最后一条线段
setcolor(COLOR::RED);
line(init_x, init_y, curr_x, curr_y); // 绘制当前线段
prev_x = curr_x;
prev_y = curr_y;
points.push_back(make_pair(curr_x, curr_y));
}
}
}
// 填充多边形内部
for (auto& polygon : polygons)
{
int size = polygon.size();
int* points_x = new int[size];
int* points_y = new int[size];
for (int i = 0; i < size; i++)
{
points_x[i] = polygon[i].first;
points_y[i] = polygon[i].second;
}
setfillcolor(COLOR::GREEN);
fillpolygon(points_x, points_y, size); // 填充多边形内部
delete[] points_x;
delete[] points_y;
}
// 将多边形坐标保存到文件中
ofstream fout("polygons.txt");
for (auto& polygon : polygons)
{
for (auto& point : polygon)
{
fout << point.first << " " << point.second << endl;
}
fout << endl;
}
fout.close();
// 从文件中读取多边形坐标并重新显示在界面上
ifstream fin("polygons.txt");
vector<pair<int, int>> points_read;
while (true)
{
int x, y;
fin >> x >> y;
if (!fin)
break;
if (x == -1 && y == -1)
{
polygons.push_back(points_read);
points_read.clear();
}
else
{
points_read.push_back(make_pair(x, y));
}
}
for (auto& polygon : polygons)
{
int size = polygon.size();
int* points_x = new int[size];
int* points_y = new int[size];
for (int i = 0; i < size; i++)
{
points_x[i] = polygon[i].first;
points_y[i] = polygon[i].second;
}
setcolor(COLOR::BLUE);
drawpolyline(points_x, points_y, size); // 绘制多边形
delete[] points_x;
delete[] points_y;
}
fin.close();
system("pause");
closegraph();
return 0;
}
```
这个程序会在窗口中绘制多边形,同时还会将绘制完成的多边形坐标保存到文件中,并且可以从文件中读取已保存的多边形坐标,重新显示在界面上。
相关推荐
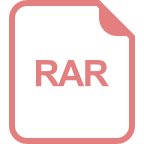
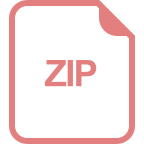














