在国际象棋的棋盘(8行x8列)上,一个马要遍历棋盘,即到达棋盘上的每一格,并且每格只到达一次。设马在棋盘的某一位置(x,y),按照“马走日” 的规则,下一步有8个方向可走,设计图形用户界面,指定初始位置(x0,y0),探索出一条或多条马遍历棋盘的路径,描绘马在棋盘上的动态移动情况。用Javaidea编写。
时间: 2024-03-01 07:50:57 浏览: 168
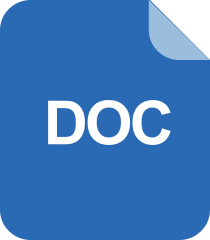
数据结构课设马踏棋盘
好的,我可以帮你回答这个问题,但是我并不是一个图形用户界面,而是一个文本交互界面。不过我可以提供一些伪代码来帮助你实现这个问题。
首先,我们需要一个棋盘对象,它可以记录每个位置是否已经被访问。我们可以用一个布尔型的二维数组来表示:
```
boolean[][] visited = new boolean[8][8];
```
接下来,我们需要一个递归函数来实现马的遍历。假设当前马的位置是(x,y),我们可以尝试从8个方向中选择一个未被访问过的位置(x',y'),然后将马移动到那里,更新visited数组,并递归调用遍历函数。如果所有的位置都已经被访问过了,那么我们就找到了一条遍历路径,可以将它记录下来。
这个遍历函数的伪代码如下:
```
void traverse(int x, int y, int step, int[][] path, List<int[][]> paths, boolean[][] visited) {
// 将当前位置加入路径
path[step][0] = x;
path[step][1] = y;
// 标记当前位置已访问
visited[x][y] = true;
// 如果已经遍历了所有位置,将路径保存下来
if (step == 63) {
paths.add(path);
return;
}
// 尝试从8个方向中选择一个未访问的位置
int[][] directions = {{-2,-1}, {-2,1}, {-1,-2}, {-1,2}, {1,-2}, {1,2}, {2,-1}, {2,1}};
for (int[] dir : directions) {
int x2 = x + dir[0];
int y2 = y + dir[1];
if (x2 >= 0 && x2 < 8 && y2 >= 0 && y2 < 8 && !visited[x2][y2]) {
traverse(x2, y2, step+1, path, paths, visited);
}
}
// 恢复当前位置的访问状态
visited[x][y] = false;
}
```
最后,我们需要一个图形用户界面来展现遍历路径。你可以使用Java Swing来实现这个界面。你可以创建一个棋盘面板,将马的位置用一个图标表示,然后在遍历过程中不断更新这个图标的位置。当遍历完成后,你可以将路径用不同的颜色绘制出来。
这个图形用户界面的伪代码如下:
```
// 创建一个棋盘面板
JPanel boardPanel = new JPanel(new GridLayout(8, 8));
for (int i = 0; i < 8; i++) {
for (int j = 0; j < 8; j++) {
JButton button = new JButton();
button.setEnabled(false);
boardPanel.add(button);
}
}
// 创建一个马的图标
ImageIcon horseIcon = new ImageIcon("horse.png");
// 遍历路径
for (int[][] path : paths) {
// 在每个位置上显示马的图标
for (int i = 0; i < 64; i++) {
int x = path[i][0];
int y = path[i][1];
JButton button = (JButton) boardPanel.getComponent(x*8+y);
button.setIcon(horseIcon);
// 暂停一段时间,让用户看清楚马的移动
Thread.sleep(100);
}
// 将路径用不同的颜色绘制出来
Graphics2D g = (Graphics2D) boardPanel.getGraphics();
g.setColor(Color.RED);
for (int i = 0; i < 63; i++) {
int x1 = path[i][0];
int y1 = path[i][1];
int x2 = path[i+1][0];
int y2 = path[i+1][1];
g.drawLine(y1*50+25, x1*50+25, y2*50+25, x2*50+25);
}
}
```
这些代码只是伪代码,你需要根据实际情况进行修改和完善。希望能够帮助到你。
阅读全文
相关推荐
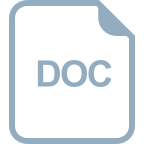
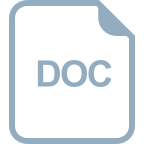


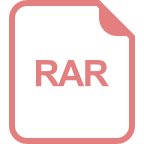
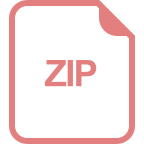
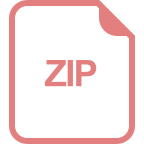
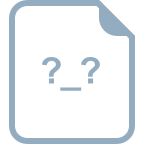
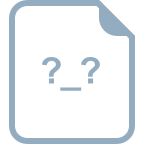







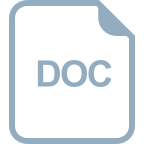
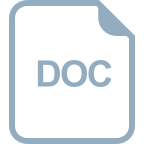