python实现数据插值
时间: 2023-10-20 21:05:57 浏览: 51
在Python中,有多种方法可以进行数据插值,以下是其中的几种:
1. 线性插值:使用scipy库的interpolate模块中的interp1d函数,该函数可以对一维数据进行线性插值。示例代码如下:
```python
from scipy.interpolate import interp1d
x = [0, 1, 2, 3, 4]
y = [0, 2, 4, 6, 8]
f = interp1d(x, y)
x_new = [1.5, 2.5, 3.5]
y_new = f(x_new)
print(y_new)
```
2. 拉格朗日插值:使用scipy库的interpolate模块中的lagrange函数,该函数可以对一维数据进行拉格朗日插值。示例代码如下:
```python
from scipy.interpolate import lagrange
x = [0, 1, 2, 3, 4]
y = [0, 2, 4, 6, 8]
f = lagrange(x, y)
x_new = [1.5, 2.5, 3.5]
y_new = f(x_new)
print(y_new)
```
3. 样条插值:使用scipy库的interpolate模块中的splrep和splev函数,该函数可以对一维数据进行样条插值。示例代码如下:
```python
from scipy.interpolate import splrep, splev
x = [0, 1, 2, 3, 4]
y = [0, 2, 4, 6, 8]
tck = splrep(x, y)
x_new = [1.5, 2.5, 3.5]
y_new = splev(x_new, tck)
print(y_new)
```
以上是三种常用的数据插值方法,根据具体情况选择合适的方法进行插值即可。
相关推荐
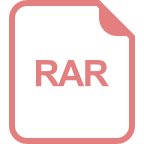














