假设二叉树每个结点的元素均为一个单字符,根据给定的字符序列按照先序遍历的顺序递归创建该树的二叉链表,然后判断该二叉树是否为二叉排序树。,用C语言写,写的简单点
时间: 2024-05-01 18:20:19 浏览: 22
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef struct TreeNode {
char val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 先序遍历创建二叉树
TreeNode* createTree(char* str, int* index) {
if (str[*index] == '#') {
(*index)++;
return NULL;
}
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = str[*index];
(*index)++;
node->left = createTree(str, index);
node->right = createTree(str, index);
return node;
}
// 判断是否为二叉排序树
bool isBST(TreeNode* root, char min, char max) {
if (root == NULL) {
return true;
}
if (root->val <= min || root->val >= max) {
return false;
}
return isBST(root->left, min, root->val) && isBST(root->right, root->val, max);
}
int main() {
char str[] = "FBD##E##A##C##";
int index = 0;
TreeNode* root = createTree(str, &index);
if (isBST(root, 'A'-1, 'Z'+1)) {
printf("该二叉树是二叉排序树\n");
} else {
printf("该二叉树不是二叉排序树\n");
}
return 0;
}
```
相关推荐
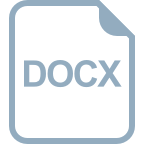
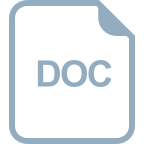
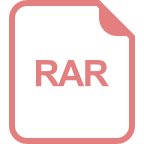








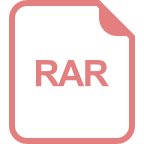
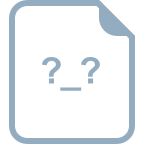
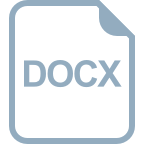
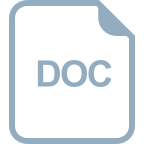
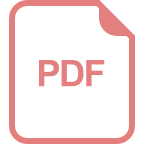
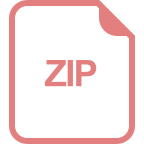
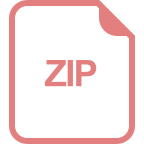