python自定义函数实现ISOMAP降维度
时间: 2024-01-01 20:53:12 浏览: 28
ISOMAP是一种流形学习的算法,用于高维数据的降维。下面是一个Python函数,可以实现ISOMAP算法的降维过程:
```python
import numpy as np
from sklearn.neighbors import NearestNeighbors
from scipy.sparse import csgraph
def isomap(X, n_components, n_neighbors):
"""
ISOMAP算法的降维过程
Parameters:
----------
X : array-like, shape (n_samples, n_features)
高维数据矩阵
n_components : int
降维后的维数
n_neighbors : int
K近邻的数量
Returns:
-------
X_iso : array-like, shape (n_samples, n_components)
降维后的数据矩阵
"""
# Step 1: 计算距离矩阵
knn = NearestNeighbors(n_neighbors=n_neighbors+1).fit(X)
dist, indices = knn.kneighbors(X)
dist = dist[:, 1:]
indices = indices[:, 1:]
# Step 2: 构造连通图
graph = np.zeros((X.shape[0], X.shape[0]))
for i in range(X.shape[0]):
graph[i, indices[i]] = dist[i]
graph[indices[i], i] = dist[i]
# Step 3: 计算最短路径距离
shortest_path = csgraph.shortest_path(graph)
# Step 4: 计算中心化距离矩阵
G = shortest_path ** 2
G -= G.mean(axis=0)
G -= G.mean(axis=1)
G += G.mean()
# Step 5: 特征值分解
eig_val, eig_vec = np.linalg.eig(G)
indices = np.argsort(eig_val)[:n_components]
eig_vec = eig_vec[:, indices]
# Step 6: 降维
X_iso = np.dot(eig_vec, np.diag(np.sqrt(eig_val[indices])))
return X_iso
```
函数中使用了scikit-learn库中的`NearestNeighbors`类来计算K近邻,使用了SciPy库中的`csgraph.shortest_path`函数来计算最短路径距离,使用了NumPy库中的`np.linalg.eig`函数来进行特征值分解。最后,根据降维后的维数和特征向量来计算降维后的数据矩阵。
使用示例:
```python
import pandas as pd
from sklearn.datasets import load_iris
import matplotlib.pyplot as plt
# 加载数据
iris = load_iris()
X = iris.data
y = iris.target
# 调用ISOMAP函数进行降维
X_iso = isomap(X, n_components=2, n_neighbors=5)
# 绘制降维后的散点图
plt.scatter(X_iso[:, 0], X_iso[:, 1], c=y)
plt.show()
```
以上代码将会对Iris数据集进行ISOMAP降维,并绘制降维后的散点图。
相关推荐
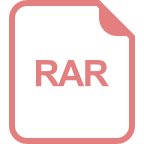







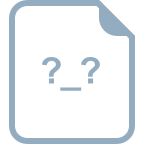
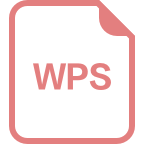
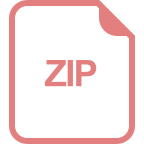
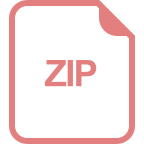