python 开发一个 A星算法接口
时间: 2023-08-10 11:05:46 浏览: 32
好的,下面是一个简单的 Python 实现 A* 算法的代码,仅供参考:
```python
from queue import PriorityQueue
# 状态类
class State:
def __init__(self, board, moves, parent=None, cost=0):
self.board = board
self.moves = moves
self.parent = parent
self.cost = cost
# 计算启发函数
def heuristic(self, goal):
count = 0
for i in range(3):
for j in range(3):
if self.board[i][j] != goal[i][j]:
count += 1
return count
# 计算总代价
def total_cost(self, goal):
return self.cost + self.heuristic(goal)
# 扩展状态
def expand(self, goal):
children = []
for i in range(3):
for j in range(3):
if self.board[i][j] == 0:
if i > 0:
new_board = self.move(i, j, i - 1, j)
children.append(State(new_board, self.moves + 'U', self, self.cost + 1).total_cost(goal))
if i < 2:
new_board = self.move(i, j, i + 1, j)
children.append(State(new_board, self.moves + 'D', self, self.cost + 1).total_cost(goal))
if j > 0:
new_board = self.move(i, j, i, j - 1)
children.append(State(new_board, self.moves + 'L', self, self.cost + 1).total_cost(goal))
if j < 2:
new_board = self.move(i, j, i, j + 1)
children.append(State(new_board, self.moves + 'R', self, self.cost + 1).total_cost(goal))
return children
# 移动
def move(self, i1, j1, i2, j2):
new_board = [[self.board[i][j] for j in range(3)] for i in range(3)]
new_board[i1][j1], new_board[i2][j2] = new_board[i2][j2], new_board[i1][j1]
return new_board
# A* 算法求解
def solve(initial, goal):
initial_state = State(initial, '', None, 0)
goal_state = State(goal, None, None)
visited = set()
pq = PriorityQueue()
pq.put((initial_state.total_cost(goal), initial_state))
while not pq.empty():
_, state = pq.get()
if state.board == goal:
return state.moves
children = state.expand(goal)
for child in children:
if str(child.board) not in visited:
pq.put((child, child.total_cost(goal)))
visited.add(str(child.board))
# 测试
if __name__ == '__main__':
initial = [[1, 2, 3], [4, 0, 6], [7, 5, 8]]
goal = [[1, 2, 3], [4, 5, 6], [7, 8, 0]]
moves = solve(initial, goal)
print(moves)
```
上面的代码中,我们定义了一个状态类 `State`,封装了状态的信息以及对状态进行操作的方法。然后,我们实现了 A* 算法的求解函数 `solve`。最后,在 `main` 函数中,我们构造了一个初始状态和目标状态,并用求解函数求解出了步骤。
相关推荐
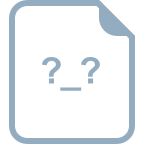
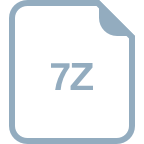














