编写一段python代码,输入平面坐标系中的两点A(X1,Y1)和B(X2,Y2),计算两点之间的距离。
时间: 2024-10-12 20:04:35 浏览: 196
当然,以下是一个简单的Python代码片段,用于计算二维平面上两个点A和B之间的欧氏距离:
```python
def distance_between_points(A, B):
# A 和 B 分别是元组,包含点的x和y坐标
x1, y1 = A
x2, y2 = B
# 欧氏距离公式:sqrt((x2-x1)^2 + (y2-y1)^2)
distance = ((x2 - x1)**2 + (y2 - y1)**2) ** 0.5
return distance
# 输入点A和B的坐标
point_A = tuple(map(int, input("请输入点A的坐标(例如:(1, 2)):").split(',')))
point_B = tuple(map(int, input("请输入点B的坐标(例如:(3, 4)):").split(',')))
distance = distance_between_points(point_A, point_B)
print(f"点A和点B之间的距离是:{distance:.2f}")
相关问题
python直角坐标系中有两个点(x1, y1)和(x2, y2),从键盘输入两个点的坐标,计算两个点之间的距离。
在Python中,两点之间的距离(Euclidean distance)可以通过勾股定理来计算,即d = sqrt((x2-x1)^2 + (y2-y1)^2)。以下是如何编写这个计算函数的示例:
```python
import math
def calculate_distance(x1, y1, x2, y2):
"""计算二维空间中两点间的欧氏距离"""
distance = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
return distance
# 从用户那里获取输入的点的坐标
x1 = float(input("请输入第一个点的x坐标:"))
y1 = float(input("请输入第一个点的y坐标:"))
x2 = float(input("请输入第二个点的x坐标:"))
y2 = float(input("请输入第二个点的y坐标:"))
# 调用函数并打印结果
distance = calculate_distance(x1, y1, x2, y2)
print(f"两点之间的距离是:{distance}.")
```
当你运行这个程序,并按照提示输入两个点的坐标,它会计算并显示这两个点之间的距离。
编写Python程序,请用户分别输入两个点坐标值:(x1,y1)及(x2,y2)。计算并输出这两点在直角坐标系中的距离,结果保留两位小数。
当然,这是一个简单的数学问题,我们可以利用Python编写一个函数来计算两点之间的欧氏距离。以下是相应的代码示例:
```python
def calculate_distance():
# 请求用户输入两个点的坐标
x1 = float(input("请输入第一个点的x坐标(x1): "))
y1 = float(input("请输入第一个点的y坐标(y1): "))
x2 = float(input("请输入第二个点的x坐标(x2): "))
y2 = float(input("请输入第二个点的y坐标(y2): "))
# 计算两点间的距离公式:sqrt((x2-x1)^2 + (y2-y1)^2)
distance = ((x2 - x1)**2 + (y2 - y1)**2)**0.5
# 保留两位小数并打印结果
formatted_distance = format(distance, '.2f')
print(f"两点之间的距离是: {formatted_distance}.")
calculate_distance()
```
运行此程序,它会提示用户输入两个点的坐标,然后计算并显示它们之间的距离。
阅读全文
相关推荐
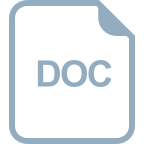
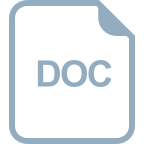
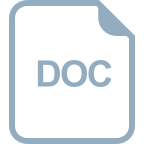













