用c语言编写1.编写程序,建立一棵二叉树(以链表存储),对该二叉树进行遍历并输出该二叉树的前序,中序,后序遍历序列(三种遍历任选一种采用非递归算法);2. 编写程序,建立一棵二叉树(以链表存储),实现二叉树左右子树的交换; 3.统计二叉树中叶子结点个数。
时间: 2024-12-03 09:14:20 浏览: 32
1. 在 C 语言中,我们可以使用结构体表示二叉树节点,并使用链表存储。首先,定义一个 `Node` 结构体:
```c
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
```
为了实现非递归的遍历,可以使用栈数据结构。以下是三种遍历方式的示例代码:
**前序遍历(根-左-右):**
```c
void preorderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s;
s.push(root);
while (!s.isEmpty()) {
TreeNode* node = s.top();
printf("%d ", node->val);
s.pop();
if (node->right) s.push(node->right);
if (node->left) s.push(node->left);
}
}
```
**中序遍历(左-根-右):**
```c
void inorderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s;
TreeNode* cur = root;
while (cur != NULL || !s.isEmpty()) {
while (cur != NULL) {
s.push(cur);
cur = cur->left;
}
cur = s.top();
s.pop();
printf("%d ", cur->val);
cur = cur->right;
}
}
```
**后序遍历(左-右-根):**
```c
void postorderTraversal(TreeNode* root) {
if (root == NULL) return;
stack<TreeNode*> s1, s2;
s1.push(root);
while (!s1.isEmpty()) {
TreeNode* node = s1.top();
s1.pop();
s2.push(node);
if (node->left) s1.push(node->left);
if (node->right) s1.push(node->right);
}
while (!s2.isEmpty()) {
printf("%d ", s2.top()->val);
s2.pop();
}
}
```
2. 实现二叉树左右子树交换:
```c
void swapLeftAndRightChild(TreeNode* node) {
if (node != NULL) {
TreeNode temp = node->left;
node->left = node->right;
node->right = temp;
}
}
```
3. 统计二叉树中叶子结点个数:
```c
int countLeaves(TreeNode* root) {
if (root == NULL) return 0;
if (root->left == NULL && root->right == NULL) return 1;
return countLeaves(root->left) + countLeaves(root->right);
}
```
阅读全文
相关推荐
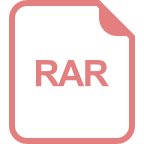
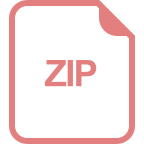
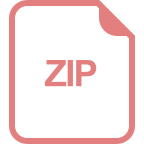














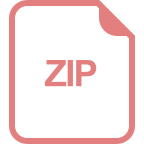
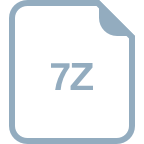