C++用一个函数计算圆形,梯形,矩形,三角形的面积
时间: 2024-09-13 13:13:34 浏览: 73
在C++中,你可以通过定义一个函数,并利用函数的参数传递不同的类型来实现计算不同图形的面积。这可以通过使用函数重载或者传递参数类型来实现。下面是一个使用继承和虚函数实现的简单示例,其中基类定义了一个纯虚函数来计算面积,派生类重写该函数来实现具体图形的面积计算。
首先,定义一个基类`Shape`,它包含一个纯虚函数`getArea()`,然后为每种图形定义一个派生类,这些类都继承自`Shape`并实现了`getArea()`方法。
```cpp
#include <iostream>
#include <cmath>
// 基类
class Shape {
public:
// 纯虚函数,用于获取图形的面积
virtual double getArea() const = 0;
virtual ~Shape() {}
};
// 圆形类
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double getArea() const override {
return M_PI * radius * radius;
}
};
// 梯形类
class Trapezoid : public Shape {
private:
double top, bottom, height;
public:
Trapezoid(double t, double b, double h) : top(t), bottom(b), height(h) {}
double getArea() const override {
return (top + bottom) * height / 2;
}
};
// 矩形类
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() const override {
return width * height;
}
};
// 三角形类
class Triangle : public Shape {
private:
double base, height;
public:
Triangle(double b, double h) : base(b), height(h) {}
double getArea() const override {
return base * height / 2;
}
};
int main() {
Circle circle(5);
Trapezoid trapezoid(3, 4, 5);
Rectangle rectangle(4, 6);
Triangle triangle(3, 4);
std::cout << "Circle area: " << circle.getArea() << std::endl;
std::cout << "Trapezoid area: " << trapezoid.getArea() << std::endl;
std::cout << "Rectangle area: " << rectangle.getArea() << std::endl;
std::cout << "Triangle area: " << triangle.getArea() << std::endl;
return 0;
}
```
在这个示例中,`getArea()`方法被重写以计算每种图形的面积。在`main()`函数中,我们创建了每种图形的对象,并调用它们的`getArea()`方法来打印出面积。
阅读全文
相关推荐
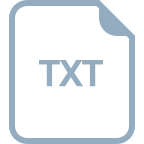
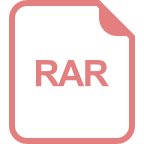
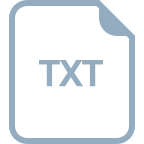















