import numpy as np from scipy.optimize import minimize from scipy.stats import norm # 定义测试函数 def test_func(t): return np.sum(t**2 - 10 * np.cos(2 * np.pi * t) + 10) # 生成200个随机数据点 np.random.seed(42) X = np.random.uniform(low=-20, high=20, size=(200, 10)) y = np.apply_along_axis(test_func, 1, X) # 定义高斯模型 class GaussianProcess: def __init__(self, kernel, noise=1e-10): self.kernel = kernel self.noise = noise def fit(self, X, y): self.X = X self.y = y self.K = self.kernel(X, X) + self.noise * np.eye(len(X)) self.K_inv = np.linalg.inv(self.K) def predict(self, X_star): k_star = self.kernel(self.X, X_star) y_mean = k_star.T @ self.K_inv @ self.y y_var = self.kernel(X_star, X_star) - k_star.T @ self.K_inv @ k_star return y_mean, y_var # 定义高斯核函数 def rbf_kernel(X1, X2, l=1.0, sigma_f=1.0): dist = np.sum(X1**2, 1).reshape(-1, 1) + np.sum(X2**2, 1) - 2 * np.dot(X1, X2.T) return sigma_f**2 * np.exp(-0.5 / l**2 * dist) # 训练高斯模型 gp = GaussianProcess(kernel=rbf_kernel) gp.fit(X, y) # 预测新数据点 X_star = np.random.uniform(low=-20, high=20, size=(1, 10)) y_mean, y_var = gp.predict(X_star) # 计算精确值 y_true = test_func(X_star) # 输出结果 print("预测均值:", y_mean) print("预测方差:", y_var) print("精确值:", y_true) print("预测误差:", (y_true - y_mean)**2) print("预测方差是否一致:", np.isclose(y_var, gp.kernel(X_star, X_star)))不能实现每次运行都是不同的结果
时间: 2024-03-16 13:47:14 浏览: 182
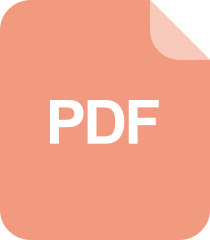
python 3.74 运行import numpy as np 报错lib\site-packages\numpy\__init__.py
如果你希望每次运行都得到不同的结果,可以在生成随机数据点时设置不同的随机数种子,例如可以用时间戳作为随机数种子:
```python
np.random.seed(int(time.time()))
X = np.random.uniform(low=-20, high=20, size=(200, 10))
y = np.apply_along_axis(test_func, 1, X)
```
这样每次运行程序时,种子都会不同,生成的随机数据点也会不同,从而得到不同的结果。
阅读全文
相关推荐
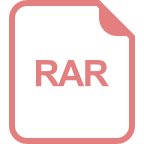



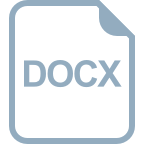
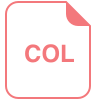
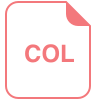
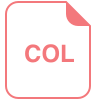
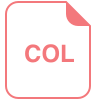
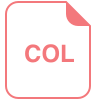
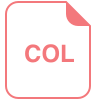
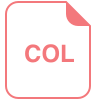
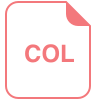
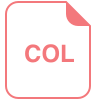
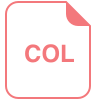
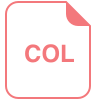
